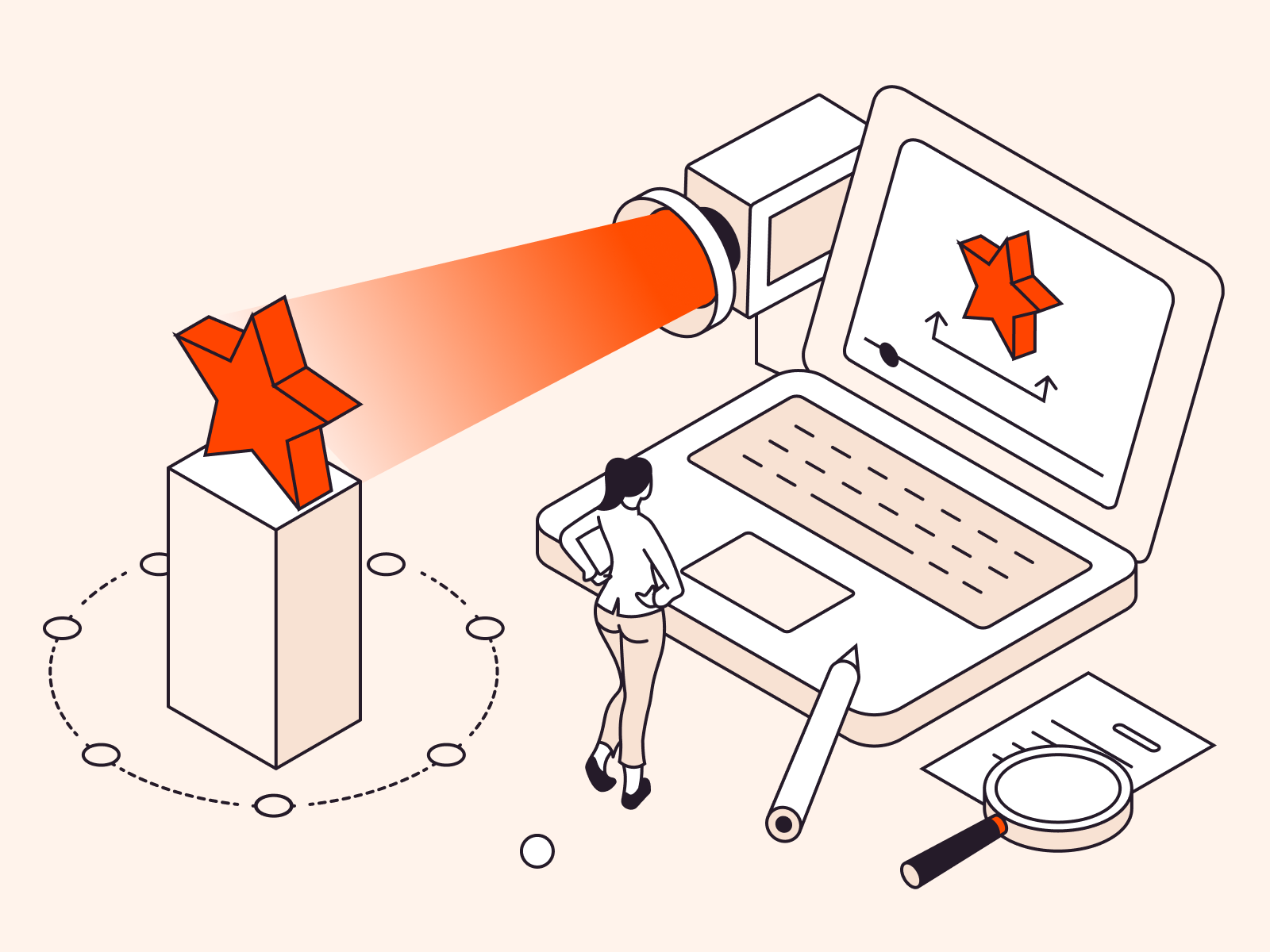
Computer vision is an innovative technology that has transformed the field of artificial intelligence, especially in video analysis. This article provides practical information on how to implement computer vision for video analysis. It offers a step-by-step guide to help you harness this powerful technology. The guide covers everything from setting up your development environment to selecting appropriate algorithms and libraries. This will help you improve your video processing capabilities significantly.
What is Computer Vision and Its Key Concepts
Computer vision is an artificial intelligence field that empowers machines to comprehend and interpret the visual world. By imitating the complexity of human vision, computer vision algorithms can recognize, categorize, and respond to elements in images and videos, converting pixels into actionable information. This technology has revolutionized various industries, from automating manufacturing processes to enhancing surveillance systems and driving the development of autonomous vehicles. Essentially, computer vision aims to bridge the gap between visual input and machine interpretation, making it a crucial element in the ongoing AI revolution.
Here are five key concepts in computer vision:
- Image Processing. The foundation of computer vision, image processing involves enhancing images (noise reduction, brightness adjustment) and preparing them (segmentation, edge detection) for further analysis.
- Feature Detection and Extraction. This involves identifying and extracting significant details from an image, such as edges, corners, or specific shapes, to facilitate object recognition or classification.
- Object Detection and Recognition. A critical aspect of computer vision, this concept focuses on identifying objects within images or videos, ranging from simple shapes to complex structures like human faces or vehicles.
- Pattern Recognition and Classification. This process categorizes visual inputs into defined groups based on their features, enabling machines to recognize patterns and make predictions about new, unseen images.
- Deep Learning and Neural Networks. These advanced algorithms, particularly convolutional neural networks (CNNs), are pivotal in achieving high accuracy in tasks such as image classification, object detection, and semantic segmentation, making them the backbone of modern computer vision applications.
How to Set Up the Development Environment for Computer Vision
Setting up a development environment for computer vision involves selecting the right hardware and software to effectively develop and run your computer vision applications. Here’s a comprehensive guide to getting your environment ready:
Hardware Requirements
- Processor (CPU). A fast CPU is beneficial for general programming and running algorithms. Look for modern multi-core processors for efficient multitasking and computation.
- Graphics Processing Unit (GPU). For deep learning aspects of computer vision, a powerful GPU is crucial. NVIDIA GPUs are highly recommended due to their CUDA support, which accelerates deep learning frameworks.
- RAM. Minimum of 8 GB is recommended; however, 16 GB or more is preferable for handling large datasets and complex algorithms.
- Storage. Solid State Drives (SSDs) are recommended for faster read/write speeds, which is beneficial when dealing with large image or video files.
Software Requirements
- Operating System. Most development tools and libraries are compatible with Linux, Windows, and macOS. Linux (Ubuntu) is often preferred for its open-source nature and support in the deep learning community.
- Programming Language. Python is the most popular language for computer vision due to its simplicity and the vast availability of libraries and frameworks.
- Integrated Development Environment (IDE). Choose an IDE or code editor that you’re comfortable with. Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebooks for Python development.
Essential Libraries and Frameworks
- OpenCV. Open Source Computer Vision Library, widely used for basic image processing tasks, feature detection, and object recognition.
- TensorFlow and Keras. For deep learning applications, TensorFlow offers comprehensive tools and resources for developing and training AI models. Keras provides a more user-friendly interface to TensorFlow.
- PyTorch. An alternative to TensorFlow, known for its flexibility and dynamic computational graph, making it a favorite for research and prototyping.
- Numpy and Matplotlib. Essential for numerical processing and data visualization, respectively.
Setting up your development environment correctly is crucial to achieving a seamless and productive workflow in computer vision projects. Having the appropriate hardware and software ready sets the stage for creating cutting-edge computer vision applications. The following section will discuss how to implement computer vision for video analysis.
Process to Implement Computer Vision for Video Analysis
Implementing computer vision for video analysis involves several steps, from setting up your environment to applying and refining computer vision models. Below is a step-by-step guide, complete with command examples and descriptions of each stage in the process.
#1 Environment Setup
First, ensure your development environment is ready. Refer to the “How to Set Up the Development Environment for Computer Vision” section for hardware and software requirements. Install Python and the necessary libraries like OpenCV, TensorFlow (or PyTorch), and Numpy.
Example command to install essential Python libraries:
pip install opencv-python tensorflow numpy matplotlib
#2 Load and Play Video
To begin processing a video, you first need to load and play it using OpenCV. This step ensures your setup can handle video files correctly.
Python code to load and play a video:
import cv2 # Load the video cap = cv2.VideoCapture('path/to/your/video.mp4') # Play the video while cap.isOpened(): ret, frame = cap.read() if not ret: print("Can't receive frame (stream end?). Exiting ...") break cv2.imshow('frame', frame) if cv2.waitKey(1) == ord('q'): break cap.release() cv2.destroyAllWindows()
Sample Output: A window that plays the loaded video file. Press ‘q’ to exit.
#3 Frame Extraction and Preprocessing
Extract frames from the video to apply computer vision techniques. Frames may need preprocessing (resizing, grayscale conversion) to reduce computational load and improve model accuracy.
Python code for frame extraction and preprocessing:
import cv2 cap = cv2.VideoCapture('path/to/your/video.mp4') while cap.isOpened(): ret, frame = cap.read() if not ret: break # Convert frame to grayscale gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Resize frame resized_frame = cv2.resize(gray, (320, 240)) # Display the processed frame cv2.imshow('Processed Frame', resized_frame) if cv2.waitKey(1) == ord('q'): break cap.release() cv2.destroyAllWindows()
#4 Apply Computer Vision Model
Use a pre-trained model or custom algorithm for object detection, face recognition, or any other task relevant to your video analysis. Here, we’ll use a simple face detection example with a pre-trained Haar Cascade model in OpenCV.
Python code for face detection in video frames:
import cv2 # Load pre-trained model face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml') cap = cv2.VideoCapture('path/to/your/video.mp4') while cap.isOpened(): ret, frame = cap.read() if not ret: break gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) faces = face_cascade.detectMultiScale(gray, 1.1, 4) # Draw rectangles around detected faces for (x, y, w, h) in faces: cv2.rectangle(frame, (x, y), (x+w, y+h), (255, 0, 0), 2) # Display the frame with detected faces cv2.imshow('Face Detection', frame) if cv2.waitKey(1) == ord('q'): break cap.release() cv2.destroyAllWindows()
Sample Output: A window that displays the video frames with detected faces highlighted by blue rectangles. Press ‘q’ to exit.
#5 Analyzing and Extracting Insights
The final step involves analyzing the detected objects or features to extract meaningful insights, such as counting objects, tracking movement, or recognizing specific actions.
This step is highly specific to your application and may involve:
- Counting detected objects across frames.
- Using tracking algorithms to follow objects or individuals.
- Analyzing frame data to detect specific actions or behaviors.
Due to the wide variety of possible analyses, this step is customized based on your project’s requirements and goals.
Conclusion
Implementing computer vision for video analysis greatly improves our ability to analyze and gain insights from video content. Gcore Video for AI takes this advancement further, offering fully managed AI video services for both VOD and live streaming. With capabilities such as AI Speech Transcription, Subtitles Translation, and Content Moderation, Gcore enhances and simplifies your video analysis projects. Ready to transform your video projects? Explore Gcore Video for AI and discover how we can help you achieve your vision.