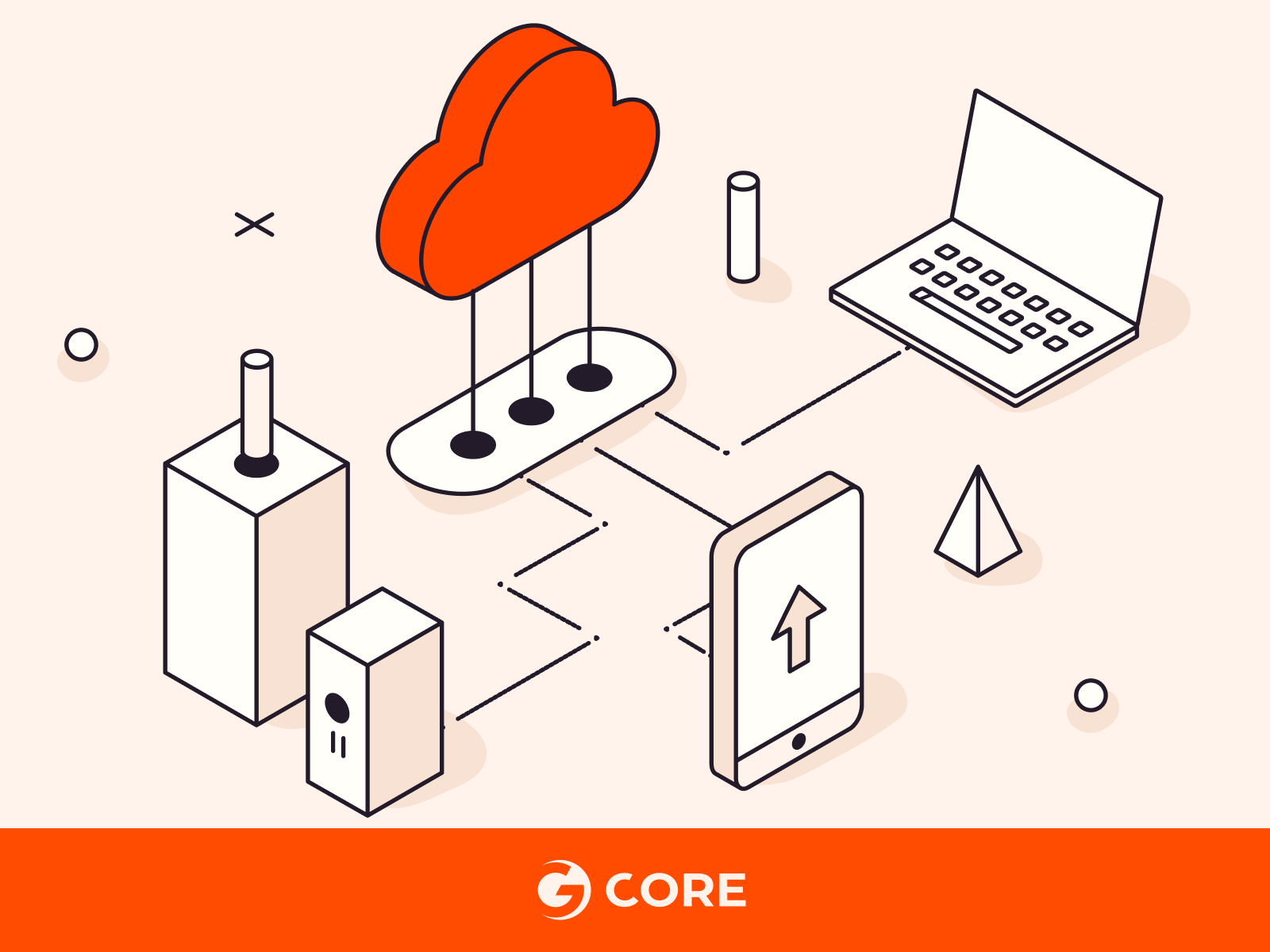
Cloud development allows you to write, debug, and run code directly in the cloud infrastructure, rather than working in the local environment and subsequently uploading to the cloud. This streamlines the development process, allowing you to deploy and update applications faster. This article explains what cloud development is, what tools can help you with it, and how you can use cloud development to develop and update your application to meet your customers’ needs.
What Is Cloud Development?
Cloud development is the practice of creating and managing applications that run on remote servers, allowing users to access them over the internet.
Every application is made up of different types of services, such as backend services, frontend services, and monitoring services. Normally, without cloud development, creating a new service or updating an existing service means writing and running your code in the local environment first. After ensuring your service works as expected, you then push your code to the cloud environment, and run it there. Finally, you publish your service and integrate it with the app. This process is time-consuming, and requires sufficiently powerful computing resources to run the service on the local machine.
This is when cloud development proves its value. With cloud development, you write your code directly in the cloud infrastructure. After you have finished writing your code, publishing your service takes just one click.
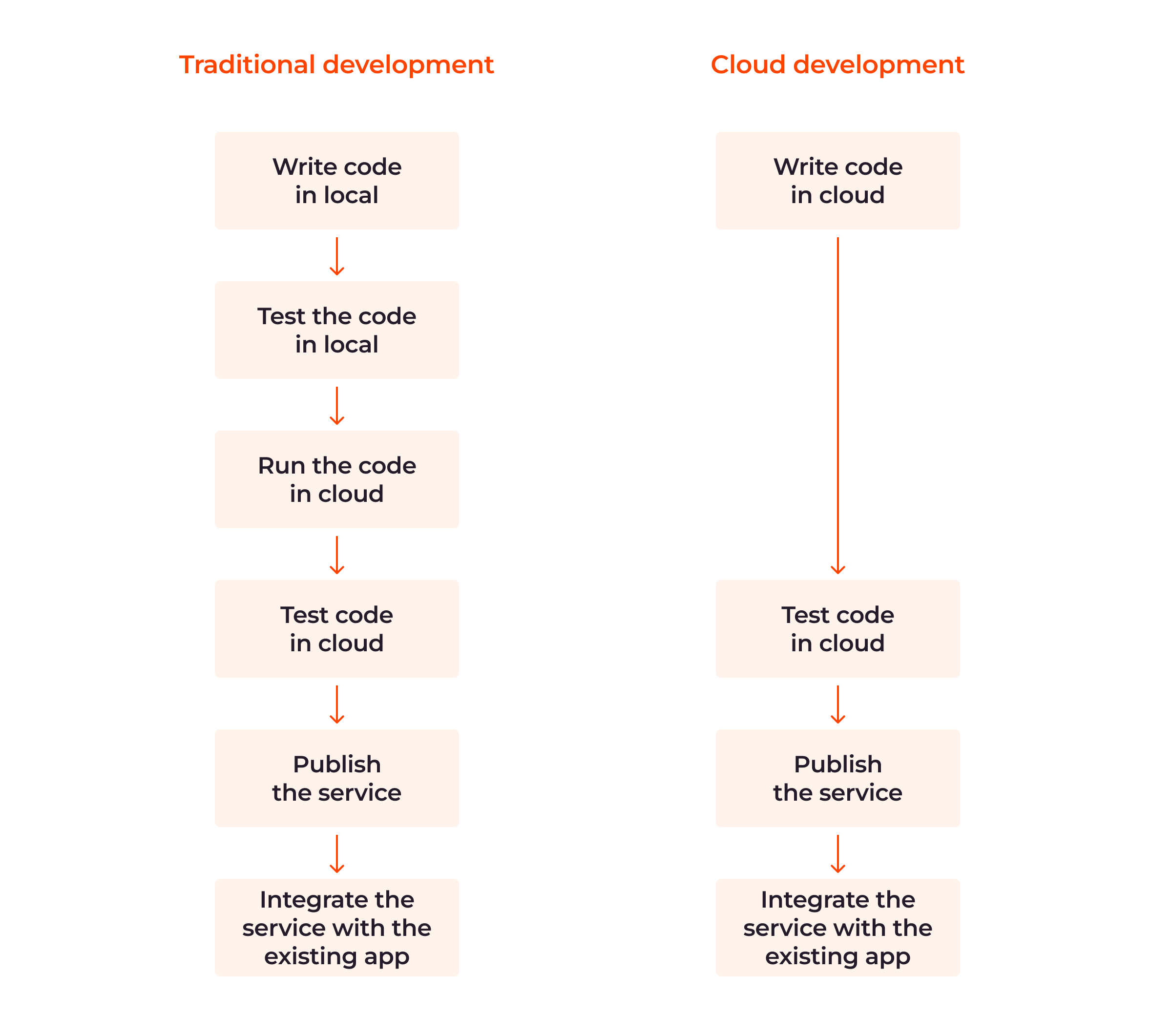
Useful Cloud Development Tools
To apply cloud development to your projects, several tools are required to build and manage the code efficiently:
- Code editor to help you write code more efficiently
- Version control tool to manage the code changes
- Compiler/interpreter to run your code
- Publisher to allow public use of your application
Let’s learn about each of the tools in more detail.
Code Editor
A code editor is a software tool that supports code highlighting, easy navigation within the codebase, test execution, and debugging capabilities. When you’re working on applications that are hosted in a cloud environment, it’s essential for your code editor to support remote access because the code you’re working on is stored on a remote cloud server.
Remote access support enables you to establish an SSH connection between your local code editor and the remote server. You can then use your code editor to create, view, and modify code files as if they were local.
Popular code editors—like Visual Studio Code and JetBrains IDE—have features that support remote development. For example, with Visual Studio Code, you can install Microsoft’s “Remote – SSH” extension to enable remote code access via SSH. Once the extension is installed, you can connect to the remote server by entering its IP address, username, and password, and work on your cloud-based projects just as easily as you would local ones.
Below is an example of using Visual Studio Code to access code via a remote machine.
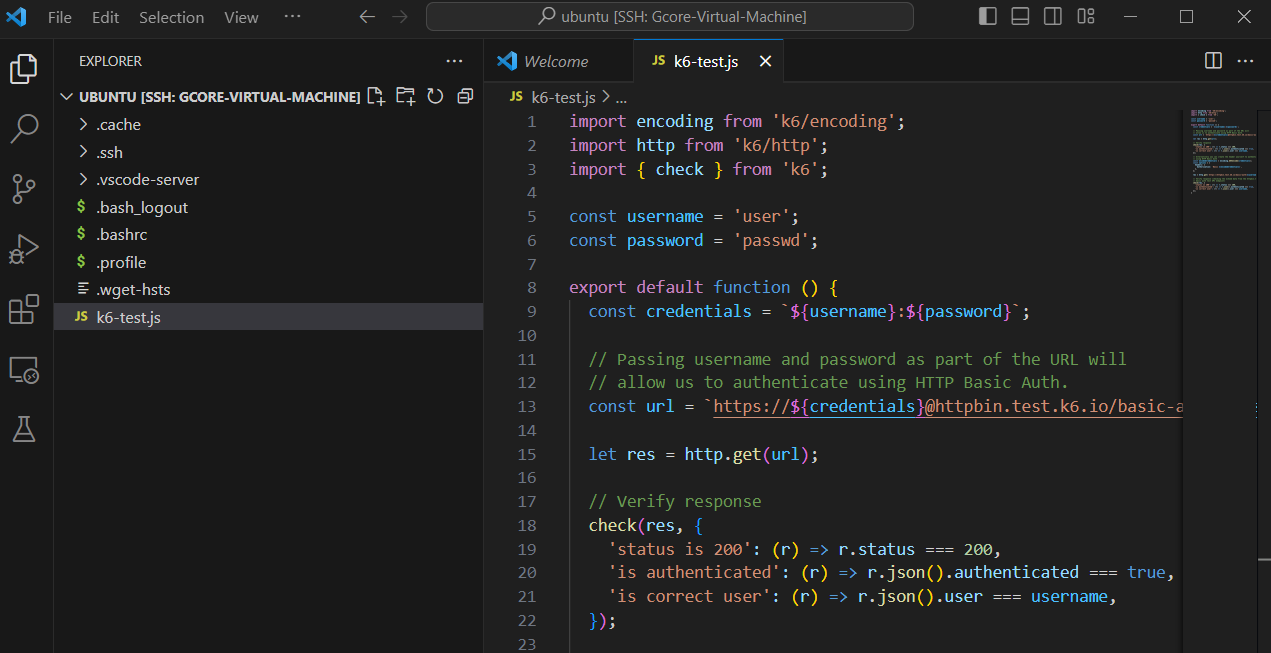
Version Control
In software development, it’s common for many people to be working on the same code base. Having a version control tool allows you to review who made the changes, and when, to certain lines of code so that you can trace any problems back to their source. Having a version control tool also allows you to revert your code to the version you want in the instance that new code introduces bugs.
There are several version control tools out there, such as Git, SVN, and Mercurial; Git is currently the most popular. Git is an open-source version control system that allows you to manage the changes in the code. It is distributed software, meaning that you create a local copy of the Git repository on your local machine, and then create new branches, add files, commit, and merge locally. When your code is ready to ship, you then push your code to the Git repository on the server.
Compiler/Interpreter
After tools that help you to write and track changes in the code, the next most important tool required to run code in the cloud is a compiler or interpreter. You need either a compiler or an interpreter to translate your code into machine code, depending on the programming languages or the runtime you are working on. They allow the computer to understand and execute your instructions. Generally speaking, the compiler or interpreter helps to build your code into executable files. Let’s look at each in turn to understand their differences.
Compiler
Before a service actually runs, a compiler translates the high-level code that you have written into low-level code. For example, a Java compiler compiles source code to the bytecode first, then the Java Virtual Machine will interpret and convert the bytecode to a machine-code executable file. As a result, compilers require time to analyze the source code. However, they also show obvious syntax errors, so you don’t need to spend a lot of time debugging your service when it’s running.
The programming languages that use compilers to translate code are Java, C#, and Go.
Interpreter
Unlike a compiler, an interpreter only translates written code into machine code when the service runs. As a result, the interpreter does not take time to compile the code. Instead, the code is executed right away. However, an application using an interpreter is often slower than one using a compiler because the interpreter executes the code line by line.
The programming languages that use interpreters are Python, Javascript, and Ruby.
Publisher
To allow other users to access your service, you need a publisher tool. This manages the following key aspects of your service:
- Configuring the network
- Creating the domain name
- Managing the scalability
Network Configuration
To allow users to access your service, the network configuration is crucial. The method for making your service available online varies based on your technology stack. For instance, if you use the Next.js framework to build your web application, you can choose Vercel to deploy your application code.
You can also customize the behavior of your application with network configuration. Here’s an example of how to use the vercel.json
file to redirect requests from one path to another:
{ "redirects": [ { "source": "/book", "destination": "/api/book", "statusCode": 301 } ] }
Domain Setting
Every service requires a URL for applications to interact with it. However, using direct IP addresses as URLs can be complex and unwieldy, so it’s advisable to assign a domain name to your service, like www.servicedomain.com. Various platforms, such as GoDaddy or SquareSpace, offer domain registration services for this purpose.
Scalability
To allow your service to handle more requests from your users, you need to define a scalability mechanism for your services. This way, your service will automatically scale according to the workload. Scalability also keeps costs in check; you pay for what you use, rather than wasting money by allocating resources based on peak usage.
Below is an example definition file for applying autoscaling to your service, using Kubernetes HorizontalPodAutoscaler.
apiVersion: autoscaling/v1 kind: HorizontalPodAutoscaler metadata: name: app spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: appdeploy minReplicas: 1 maxReplicas: 10 targetCPUUtilizationPercentage: 70
How to Run Code In the Cloud
Now that you are familiar with the tools you need for cloud development, let’s learn about how to run code in the cloud. There are two ways to run code in the cloud: using virtual machines or using containers. We explain the difference in depth in our dedicated article, but let’s review their relevance to cloud development here.
Virtual Machines
A virtual machine (VM) is like a computer that runs within a computer. It mimics a standalone system, with its own virtual hardware and software. Since a VM is separate from its host computer, you can pick the VM operating system that suits your needs without affecting your host’s system. Plus, its isolation offers an extra layer of security: if one VM is compromised, the others remain unaffected.
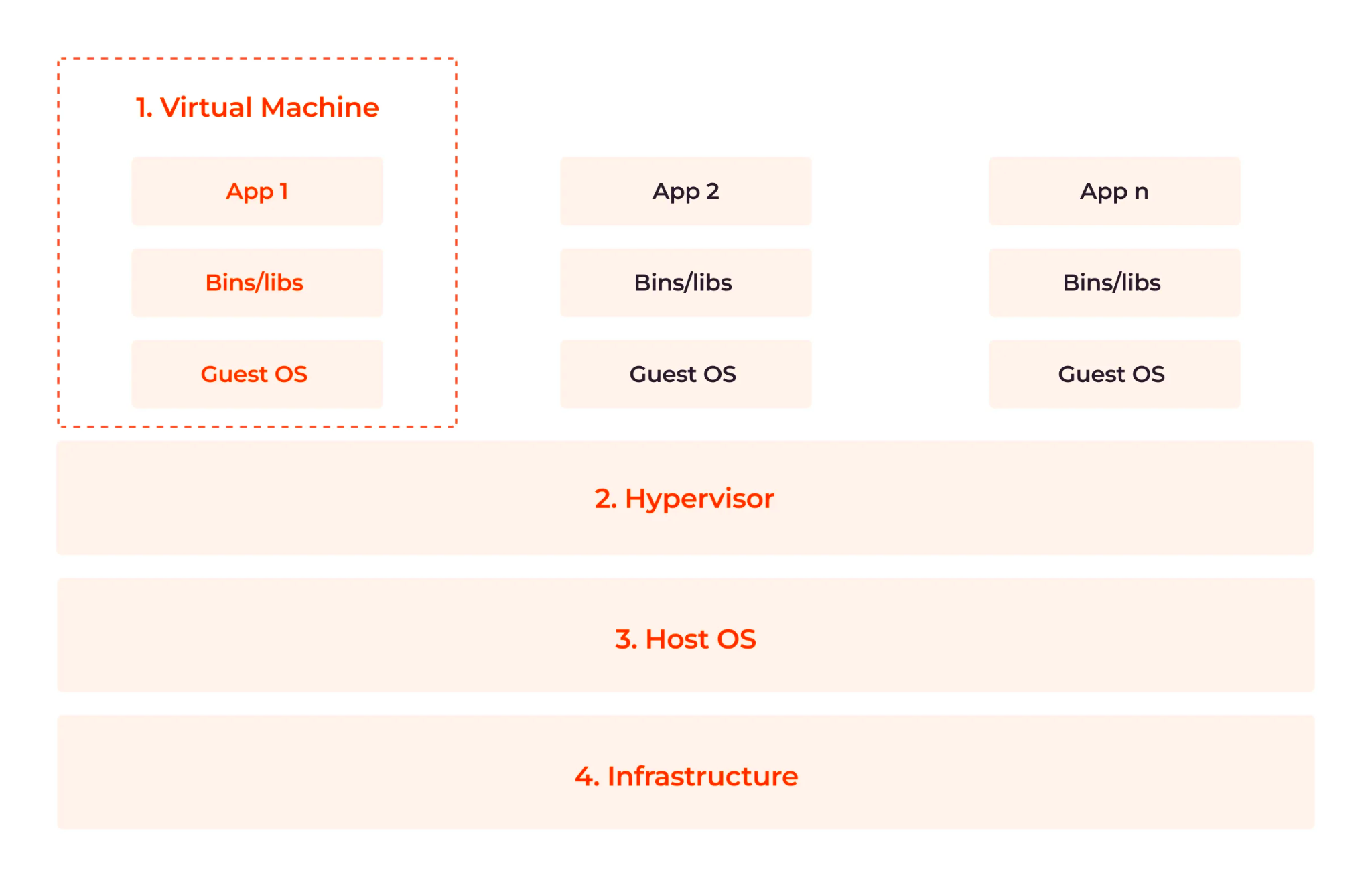
While VMs offer versatility in terms of OS choices for cloud development, scaling applications on VMs tends to be more challenging and costly compared to using containers. This is because each VM runs a full operating system, leading to higher resource consumption and longer boot-up times. Containers, on the other hand, share the host OS and isolate only the application environment, making them more lightweight and quicker to scale up or down.
Containers
A container is a software unit that contains a set of software packages and other dependencies. Since it uses the host operating system’s kernel and hardware, it doesn’t possess its own dedicated resources as a virtual machine does. As a result, it’s more lightweight and takes less time to start up. For instance, an e-commerce application can have thousands of containers for its backend and frontend services. This allows the application to easily scale out when needed by increasing the number of containers for its services.
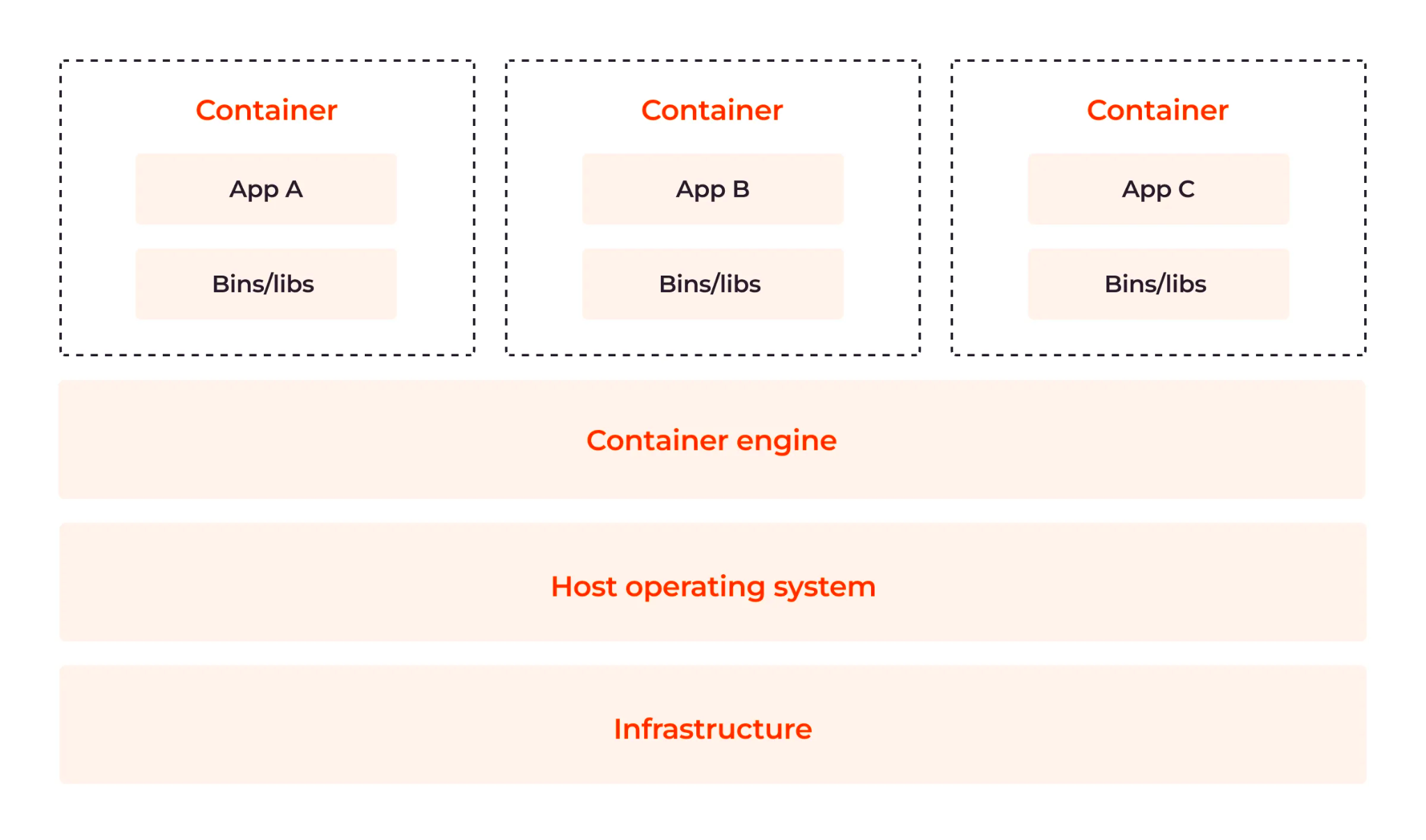
Using containers for cloud code enables efficient resource optimization and ease of scaling due to their lightweight nature. However, you have limited flexibility in choosing the operating system, as most containers are Linux-based.
Cloud Development Guide
We’ve addressed cloud development tools and ways to run code in the cloud. In this section, we offer a step-by-step guide to using cloud development for your project.
Check Computing Resources
Before anything else, you’ll need the correct computing resources to power your service. This includes deciding between virtual machines and containers. If your service tends to have a fixed number of user requests everyday, or it needs a specific operating system like Mac or Windows in order to run, go with virtual machines. If you expect your service to experience a wide range in the number of user requests and you want it to be scalable to optimize operational costs, go with containers.
After choosing between virtual machines and containers, you need to allocate computing resources for use. The important resources that you need to consider are CPUs, RAM, disk volumes, and GPUs. The specifications for these resources can vary significantly depending on the service you’re developing. For instance, if you’re building a monitoring service with a one-year data retention plan, you’ll need to allocate disk volumes of approximately 100GB to store all generated logs and metrics. If you’re building a service to apply deep learning models to large datasets, you’ll require not only a powerful CPU and ample RAM, but also a GPU.
Install Software Packages and Dependencies
After preparing the computing resources, you’ll next install the necessary software and dependencies. The installation process varies depending on whether you’re using virtual machines or containers.
As a best practice, you should set up the mechanism to install the required dependencies automatically upon initialization of the virtual machine or container. This ensures that your service has all the necessary dependencies to operate immediately upon deployment. Additionally, it facilitates easy redeployment to a different virtual machine or container, if necessary. For example, if you want to install software packages and dependencies for an Ubuntu virtual machine to host a Node.js service, you can configure cloud-init scripts for the deployed virtual machine as below:
#cloud-config ... apt: sources: docker.list: source: deb [signed-by=$KEY_FILE] https://deb.nodesource.com/node_18.x $RELEASE main keyid: 9FD3B784BC1C6FC31A8A0A1C1655A0AB68576280 package_update: true package_upgrade: true packages: - apt-transport-https - ca-certificates - gnupg-agent - software-properties-common - gnupg - nodejs power_state: mode: reboot timeout: 30 condition: True
To set up a web service on containers using Node.js, you’ll need to install Node along with the required dependencies. Below is a Dockerfile example for doing so:
# Pull the Node.js image version 18 as a base image FROM node:18 # Set the service directory WORKDIR /usr/src/app COPY package*.json ./ # Install service dependencies RUN npm install
Write Code
When you’ve installed the necessary software packages and dependencies, you can begin the fun part: writing the code. You can use code editors that support remote access to write the code for your service directly in the cloud. Built-in debugging tools in these editors can help you to identify any issues during this period.
Below is an example of using IntelliJ to debug a Go service for managing the playlists.
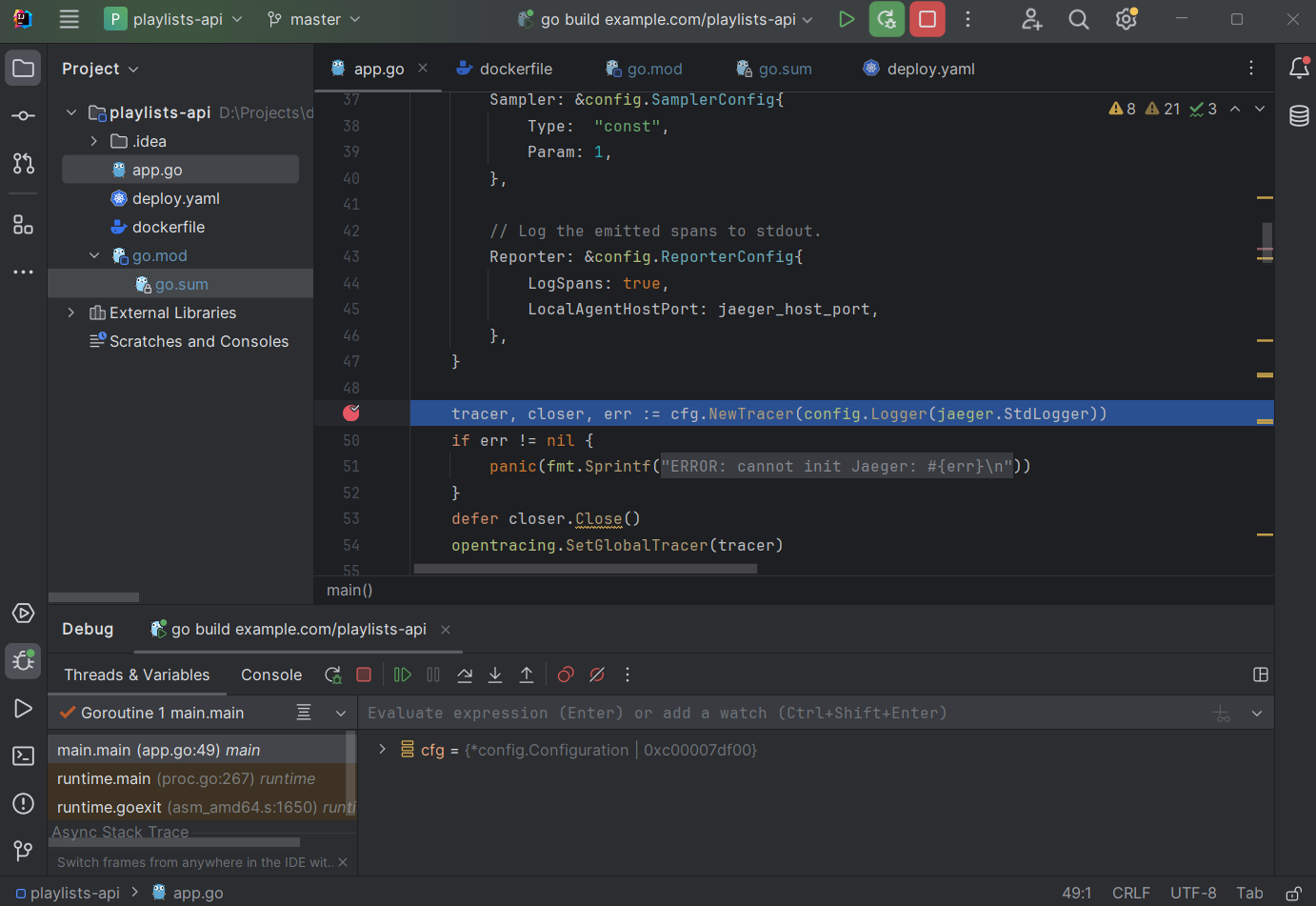
Test Your Service
After you finish writing your code, it’s crucial to test your service. As a best practice, start with unit tests to ensure that individual components work, followed by integration tests to see how your service interacts with existing application services, and finally E2E (end-to-end) tests to assess the overall user experience and system behavior.
Below is a test pyramid that gives a structured overview of each test type’s coverage. This will help you allocate your testing efforts efficiently across unit and integration tests for your service.
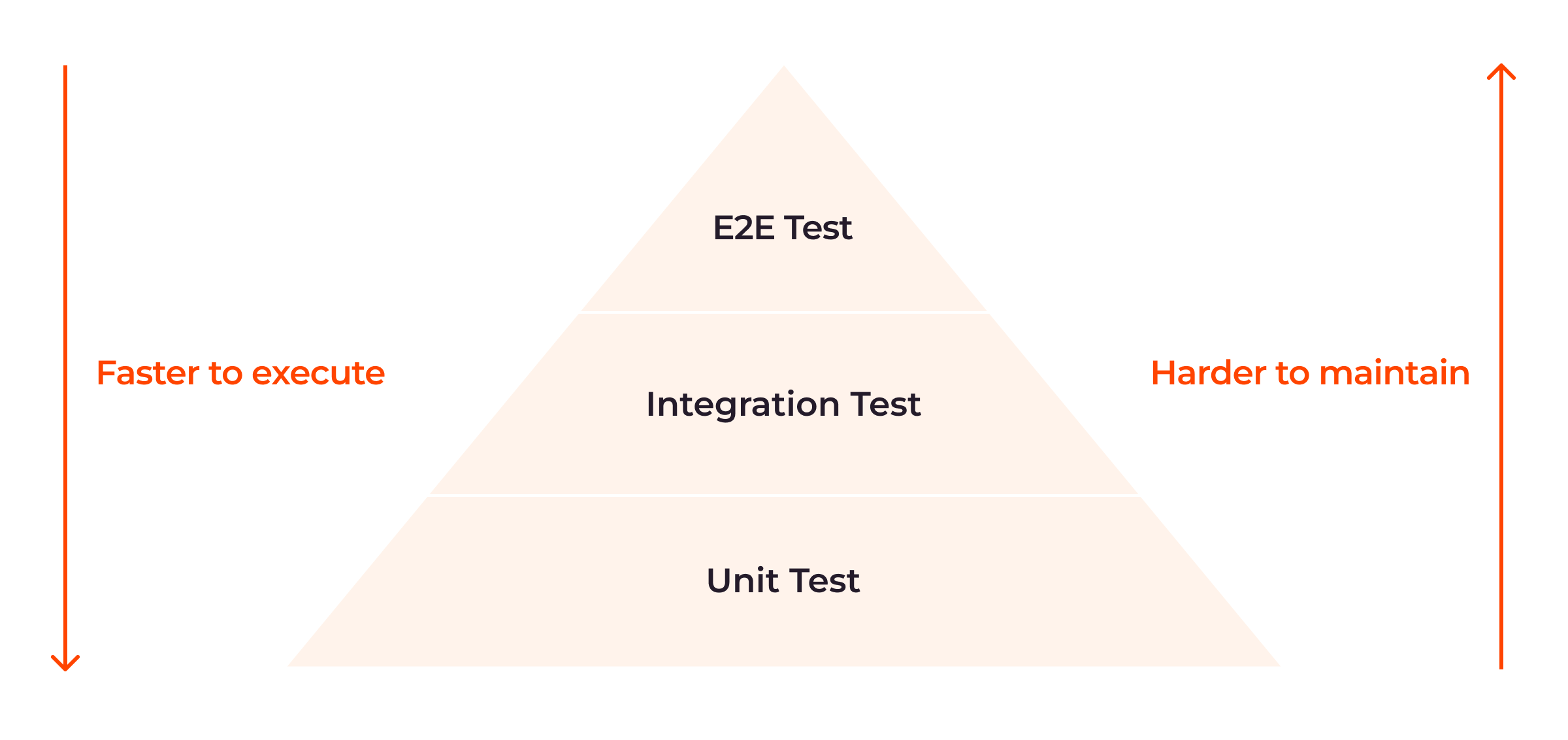
Configure Network Settings
To make your service available to users, you need to configure its network settings. This might involve configuring the rules for inbound and outbound data, creating a domain name for your service, or setting a static IP address for your service.
Here is an example of using cloud-init configuration to set a static IP for a virtual machine that hosts your service:
#cloud-config ... write_files: - content: | network: version: 2 renderer: networkd ethernets: enp3s0: addresses: - 192.170.1.25/24 - 2020:1::1/64 nameservers: addresses: - 8.8.8.8 - 8.8.4.4 path: /etc/netplan/00-add-static-ip.yaml permissions: 0644 power_state: mode: reboot timeout: 30 condition: True
Add Autoscaling Mechanism
With everything in place, it’s time to add an autoscaling mechanism. This adjusts resources based on demand, which will save costs during quiet times and boost performance during busy periods.
Assuming that you use Kubernetes to manage the containers of your service, the following is an example of using Gcore Managed Kubernetes to set the autoscaling mechanism for your Kubernetes cluster:
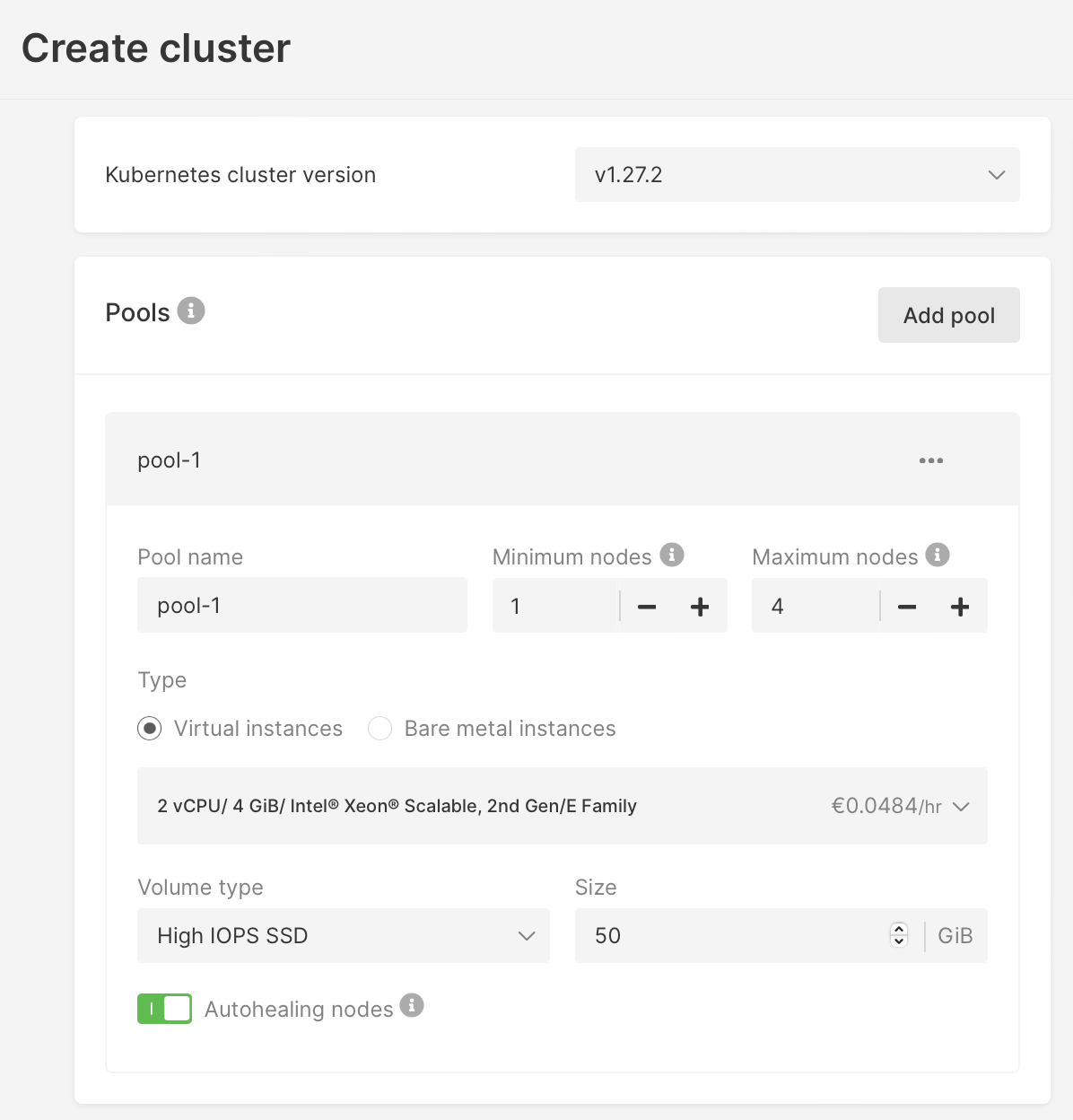
Set Up Security Enhancement
Finally, ensure your service is secure. Enhancements can range from setting up robust authentication measures to using tools like API gateways to safeguard your service. You can even set up a mechanism to protect your service from malicious activities such as DDoS attacks.
Below is an example of how to apply security protection to your service by creating a resource for your service URL using Gcore Web Security:
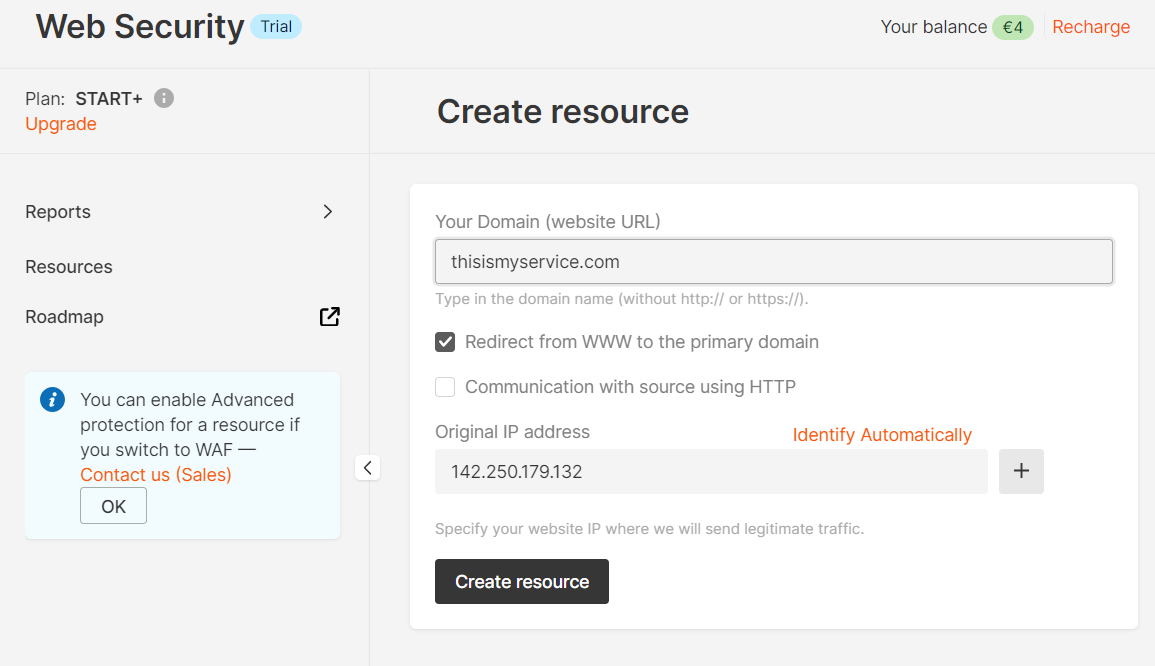
Gcore Cloud Development
Accelerating feature delivery through cloud development can offer a competitive edge. However, the initial setup of tools and environments can be daunting—and mistakes in this phase can undermine the benefits.
Here at Gcore, we recognize these obstacles and offer Gcore Function as a Service (FaaS) as a solution. Gcore FaaS eliminates the complexities of setup, allowing you to dive straight into coding without worrying about configuring code editors, compilers, debuggers, or deployment tools. Ideally suited for straightforward services that require seamless integration with existing applications, Gcore FaaS excels in the following use cases:
- Real-time stream processing
- Third-party service integration
- Monitoring and analytics services
Conclusion
Cloud development allows you to deliver your service to users immediately after you’ve finished the coding and testing phases. You can resolve production issues and implement features faster to better satisfy your customers. However, setting up cloud infrastructure can be time intensive and ideally needs a team of experienced system administrators for building and maintenance.
With Gcore FaaS, you don’t have to take on that challenge yourself. You can focus on writing code, and we’ll handle the rest—from configuring pods and networking to implementing autoscaling. Plus, you are billed only for time your customers actually use your app, ensuring cost effective operation.
Want to try out Gcore FaaS to see how it works? Get started for free.