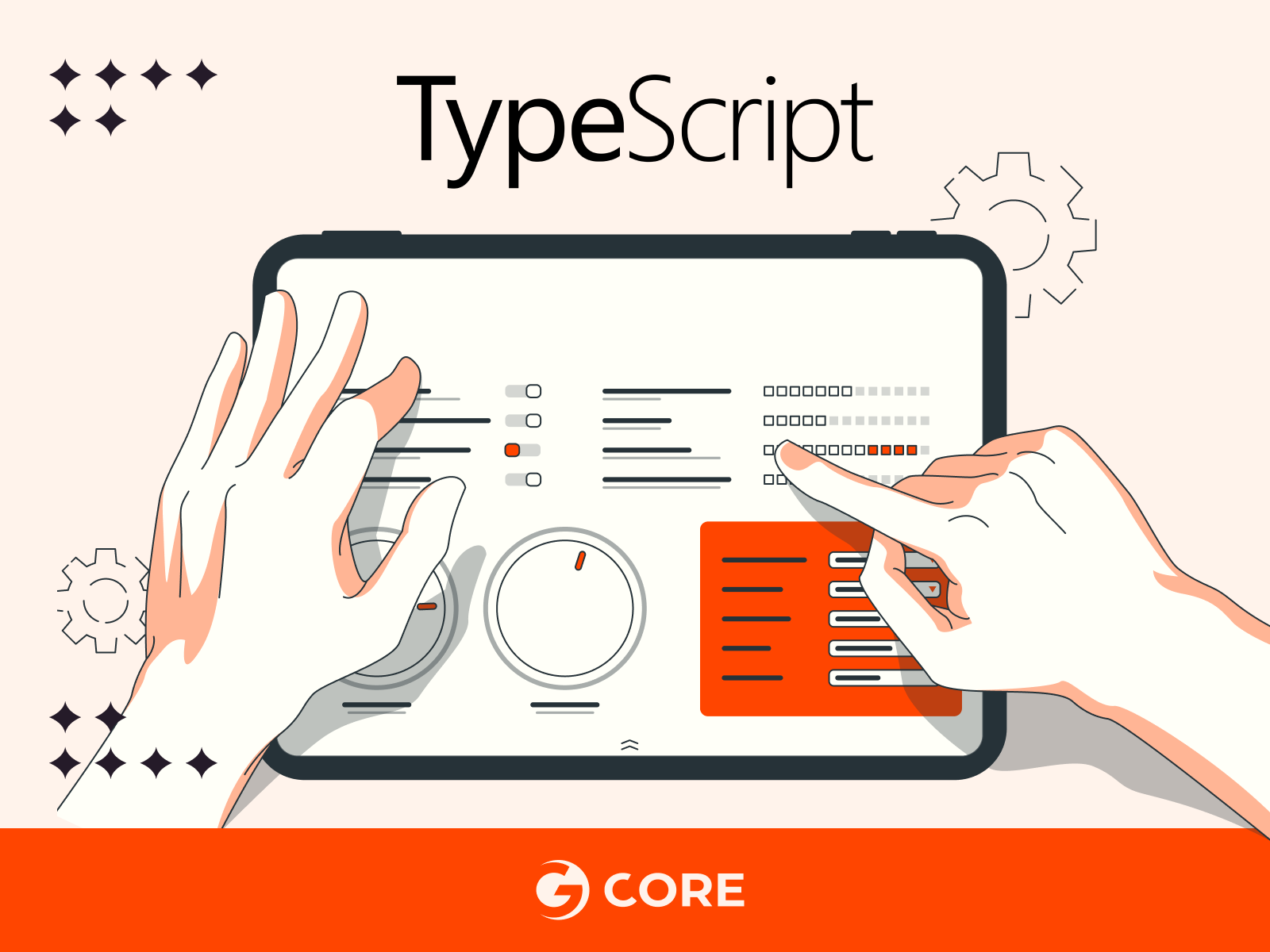
The tsconfig.json file is a powerful tool that allows you to customize the behavior of the TypeScript compiler to suit your projectâs specific needs. By tweaking the compiler options in this configuration file, you can tailor TypeScript to your projectâs requirements, enabling better control over type checking, module resolution, and compilation targets. In this article, weâll explore how to customize the tsconfig.json file and harness its potential for your TypeScript projects.
Understanding the tsconfig.json File Structure
Before we dive into customization, letâs familiarize ourselves with the structure of the tsconfig.json file. This file is written in JSON format and contains a set of key-value pairs representing different compiler options. Each option modifies a specific aspect of the TypeScript compilation process, such as target version, module system, and strictness.
Step 1: Create a New TypeScript Project
First, create a new directory for your project and navigate into it using a terminal or command prompt. Use the following command to initialize a new TypeScript project:
npm init -y
This command initializes a new npm project with default settings, creating a package.json file in the process.
Step 2: Install TypeScript
Next, install TypeScript as a development dependency by running the following command:
npm install typescript --save-dev
This command installs the TypeScript compiler and adds it as a devDependency in your package.json file.
Step 3: Generate the tsconfig.json File
To generate the tsconfig.json file with default settings, use the TypeScript compiler command-line interface (CLI) with the tsc –init command:
npx tsc --init
This command creates a new tsconfig.json file in your projectâs root directory.
Step 4: Configure Compiler Options
Customizing Compiler Options To customize the tsconfig.json file, open it in a text editor and modify the compiler options based on your projectâs requirements. Here are some commonly customized options:
- target: The target option specifies the ECMAScript version to which your TypeScript code will be compiled. It is essential to set the target version compatible with your projectâs deployment environment. Common values include âes5,â âes6,â âes2015,â or âesnext.â
- module: The module option determines the module system used in your TypeScript code. It specifies how the compiled JavaScript modules are generated and consumed. Common module options are âcommonjs,â âamd,â âes2015,â and âesnext.â The choice of module system depends on your projectâs target platform or module bundler requirements.
- outDir: The outDir option specifies the output directory for compiled JavaScript files. It defines the location where the TypeScript compiler places the transpiled JavaScript files. By default, it is set to â./distâ. Adjust this option according to your projectâs directory structure.
- rootDir: The rootDir option designates the root directory of your TypeScript source files. It tells the compiler where to start looking for TypeScript files. By default, it is set to â./srcâ, assuming your source files are located in a folder named âsrc.â Modify this option to match the correct directory structure of your project.
- strict: The strict option enables strict type-checking options in TypeScript. When set to true, TypeScript enforces stricter type checking rules, which helps catch potential errors during compilation. It includes several sub-options like ânoImplicitAny,â âstrictNullChecks,â âstrictFunctionTypes,â and others. Enabling strict mode is highly recommended for writing safer and more robust code.
- esModuleInterop: The esModuleInterop option simplifies the interoperability between TypeScript and CommonJS modules. When set to true, it allows you to use default imports and exports with CommonJS modules. This option is particularly useful when working with libraries that use default exports.
- sourceMap: The sourceMap option generates corresponding sourcemap files during compilation. Sourcemaps enable you to debug the original TypeScript code in the browser or development tools, even though it has been transpiled into JavaScript. Enabling sourcemaps is valuable for maintaining a smooth debugging experience, especially in large projects.
- noUnusedLocals and noUnusedParameters: These options, when set to true, flag unused local variables and parameters during compilation. They help identify unused code and encourage code cleanup. Enabling these options ensures that your codebase remains clean and free from unnecessary variables or parameters.
- strictNullChecks: The strictNullChecks option enforces strict null checking in TypeScript. When enabled, TypeScript detects potential null or undefined values and provides type checking to prevent errors. This option encourages safer coding practices by reducing the occurrence of null-related runtime errors.
- baseUrl and paths: These options work together to configure module resolution in TypeScript. The baseUrl option specifies the base directory for resolving module names, while the paths option allows you to define custom module name mappings. This feature is particularly useful when working with complex module structures or when using module bundlers like webpack.
Step 5: Include and Exclude Files
The tsconfig.json file allows you to specify which files to include or exclude from the compilation process. Look for the âincludeâ and âexcludeâ options and adjust them accordingly.
For example, if your source files are located in the âsrcâ folder, you can set the âincludeâ option like this:
"include": [ "src/**/*.ts" ]
This pattern includes all TypeScript files in the âsrcâ folder and its subdirectories.
Step 6: Save and Build Your Project
Once youâve configured the tsconfig.json file, save it and run the TypeScript compiler to build your project. Use the following command:
npx tsc
The TypeScript compiler will read the tsconfig.json file and compile your TypeScript code into JavaScript based on the specified settings.
Conclusion
In conclusion, configuring the tsconfig.json file is an important process in TypeScript projects because it provides precise control over the compilerâs behavior. Personalizing options according to your projectâs specific needs lets you develop more efficient, robust, and error-free code. By following the steps provided in this blog post, you can effectively harness the power of the tsconfig.json file, taking your TypeScript projects to the next level. Happy coding!