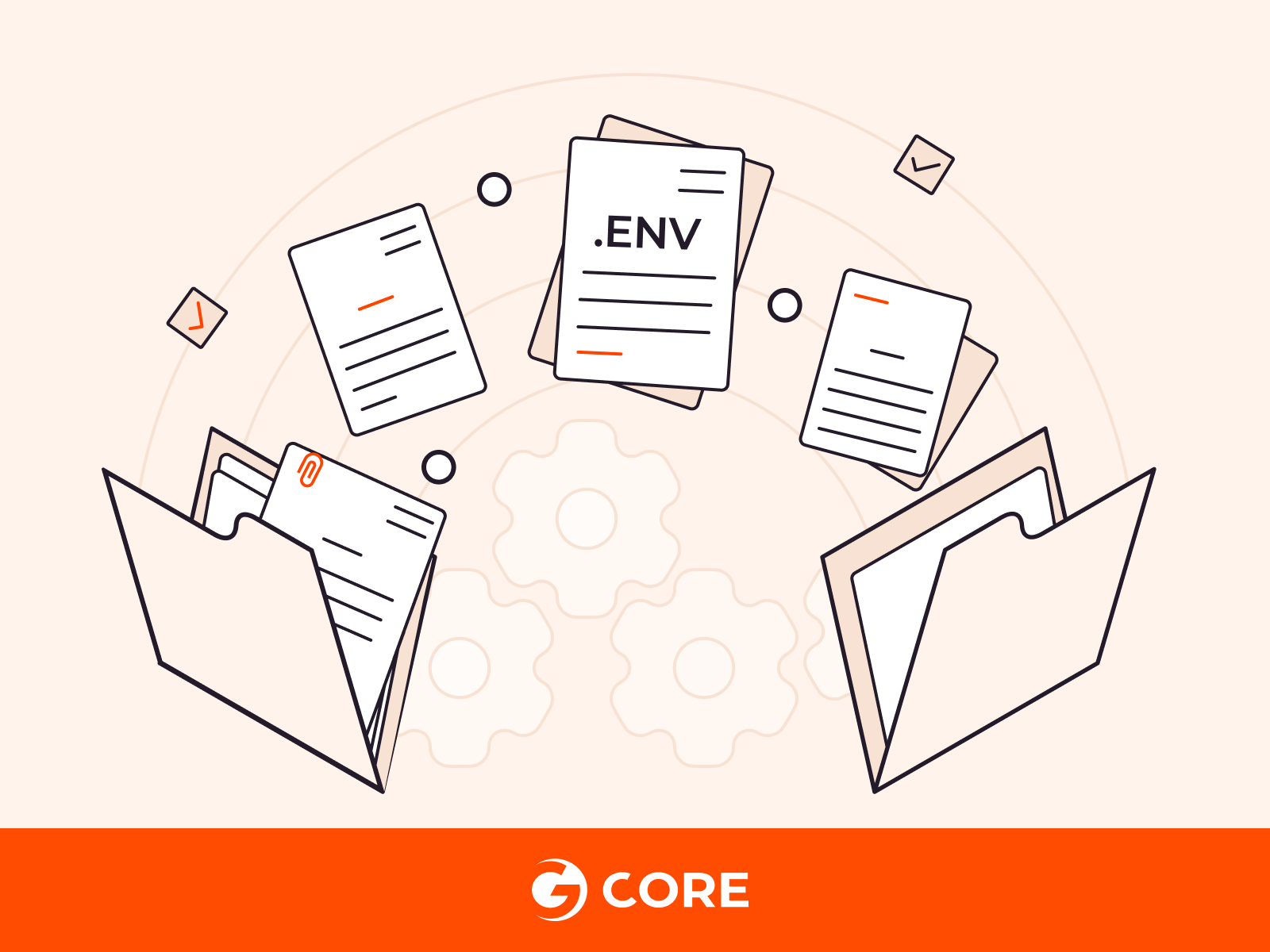
If your work involves programming or scripting, you’re familiar with the concept of variables—symbolic names that store a reference to a piece of data. Variables can be, for example, a number or a text snippet that is stored in memory. When multiple scripts or programs must have access to the same values, environment variables come into play: They are set outside of the program and shared by multiple applications. This article will explain everything you need to know about environment variables in order to set and use them effectively.
What Are Environment Variables?
Unlike regular variables, which are defined inside a program and only accessible from within its source code, environment variables are a part of the outer process where the executed software and other programs run. The process in question is usually a user session in an operating system or a virtual machine. Environment variables defined in the process are available to all programs and scripts that run inside the process.
Variables defined by a process are available to all programs and scripts that run in the process. If a process spawns a subprocess, the latter inherits all environment variables of the parent process.
Environment variables are widely used in custom scripts like shell scripts and small user programs that are executed from a command line. To name a few examples, the variables can store a path to a configuration file common to multiple apps, a URL used in a script, or a password that is used by a program but can’t be stored within that program’s source code due to security reasons.
When Are Environment Variables Used?
The most common use cases for environment variables are:
- Shared parameters or input data
- Secret values that should not be stored inside the source code
- Decoupling input data from the program to minimize a need in redeploying the application
- Using data provided by the operating system
In the first three cases, variables are set by the user—we’ll see exactly how this is done later (under “How to Set Environment Variables”)—while in the fourth case, variables are provided by the operating system. Let’s now look at a few examples of the latter.
Examples of Environment Variables
Executing scripts often requires data provided by the operating system. For example, advanced macOS and Linux users are familiar with the PATH
variable, which stores a list of locations in the file system where the operating system looks for installed command line tools. When you install a command line application like Git, a software version control system, and execute one of its commands—for example, git status
—the system checks locations stored in the PATH variable to determine where exactly the application associated with the command is located.
Another common example of an environment variable is JAVA_HOME
, which is used in both Windows- and UNIX-families of operating systems. When you install Java Development Kit (JDK,) it’s common to add the JAVA_HOME
variable to the system environment variables to let other installed software know where to find the installed copy of JDK.
Types of Environment Variables
In Windows, environment variables can be divided into three groups:
- System environment variables that are global to the operating system. For example,
%WINDIR%
stores a path to the directory where the Windows system is installed. - User environment variables are set by the user, stored in the user registry, and are available exclusively to processes and apps that are started by this user.
- Process environment variables are set by a running process and stay local to that process and all its child processes.
On operating systems like Linux and macOS, there are only two groups of environment variables:
- Global variables that are accessible by all processes.
- Local variables (also known as shell variables) that are available only from the terminal session in which the variable was defined.
Apart from classification based on the scope of the variable, environment variables can be divided into true- and pseudo-environment variables. True variables are regular values stored on the disk, but the other group of variables are effectively expressions; they return newly computed values each time the variable is addressed. Examples of pseudo-variables are %DATE%
which returns the current date in Windows or $RANDOM
which, in UNIX shells, always returns a new random 16-bit integer.
How to Set Environment Variables
Different operating systems use different kinds of shells in their terminals, which in turn use different sets of commands. Here are the commands for using environment variables on the most popular operating systems.
Windows
On Windows, there are two ways of setting a new environment variable. The first is through the system properties dialog; on Windows 11, it can be found by opening Settings, then clicking About → Advanced system settings → Environment Variables… In the window that appears, click New… and enter the name and value of the environment variable. There are two groups of variables in this menu: user variables and system variables.
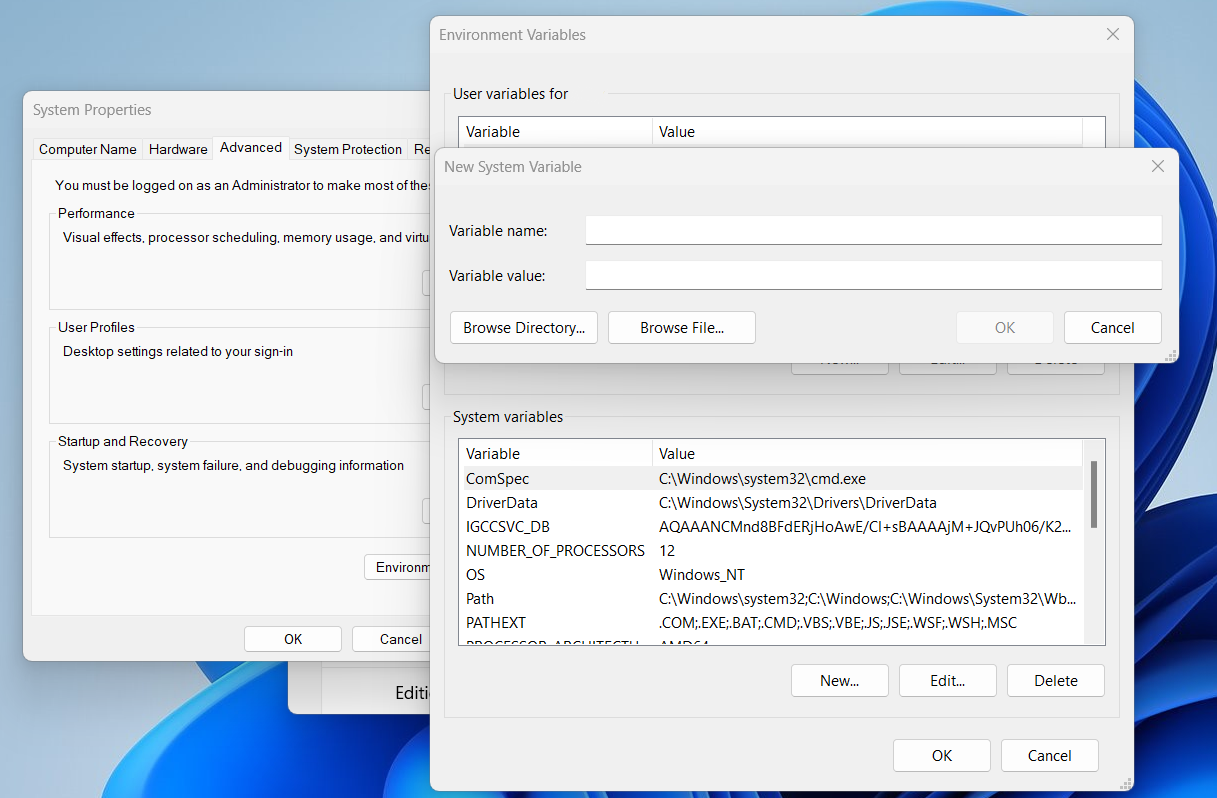
Another way of setting a variable is through the command-line interface. Here is the command:
set varName=value
varName is the variable name and value
is its value. The value in this case can only be of the text type.
To remove the variable, use the same command but without a value:
set varName=
To use a number or a numeric expression as a value, the command is slightly different:
set /a varName=31337
A variable that is set
with the set
command is temporary, visible only to the current terminal window, and is removed from memory once the window is closed. To set a variable permanently, another command is used:
setx varName value
UNIX Family
On Linux, macOS, and other operating systems of the UNIX family, environment variables are set by the following command in the terminal:
varName=value
If value
contains any whitespace, the value must be enclosed by double quotes:
varName="another value"
To remove the variable, you can set an empty value to it or use another command: unset
. Both these commands lead to the identical result:
varName=
unset varName
It’s also useful to know about a slight modification of the previous command that can be used to add a value to the current value of an existing environment variable. For example, this command adds value to the end of the current value of the varName
variable:
varName=value:$varName
To make the variable available from other terminal sessions, the keyword export
must be added in front of the main command. For example:
export varName=value
However, environment variables on UNIX systems are temporary by default. In order to make a variable outlive the current terminal session, a special command must be added to the shell startup script. Let’s do that now.
Modern versions of macOS use zsh as the default shell, so let’s use that in our example.
- Open the “zshrc” file using any available text editor, for instance, vim (in other shells, the file name will be different):
vim ~/.zshrc
- Add a command that sets an environment variable to the end of the file:
export varName=value
To start using the new variable immediately, without restarting the terminal, you need to “refresh the shell,” i.e., re-run its startup scripts. Here’s the command for refreshing zsh:
source ~/.zshrc
.env Files
In container environments like Docker, as well as common frameworks like Node.js, there’s another popular way of sharing common variables—environment or “.env” files. These are lists of key-value pairs representing variable names and their values. Docker and other deployment systems can usually read such files, and automatically load their contents into memory so that you can use them in code.
How to See the Values of Environment Variables
Sometimes, you want to check the values of environment variables before using them. Here are instructions on how to do that on different operating systems.
Windows
On Windows, apart from the GUI method of dealing with the list of environment variables described in the section “How to Set Environment Variables,” the set
command can be used. It displays the full list of currently available environment variables.
To check the value of one particular variable, the name of the variable must be specified after the command:
set varName
UNIX Family
On Linux/macOS, the command for printing out all variables is printenv
. To print a single value, the command is the following:
echo $varName
If you’re not fluent with UNIX shells, this command might look weird to you. Here’s what it means. echo
is a command for printing virtually anything to a console. Variable values in UNIX shells are referred to with the $ sign before a name, hence $varName
. Without the dollar sign, “varName” would be interpreted as a plain text instead of a variable name. Putting two parts together, we have echo $varName
.
How to Use Environment Variables
Finally, let’s look at how you can use environment variables in your programs. All programming languages and frameworks have their own means of accessing values of environment variables. For example, Python has a module called “os” which provides functions and attributes for interacting with the functionality specific to the operating system.
Here’s a simple Python function that prints a value of an environment variable called USERNAME
:
import os def print_username(): username = os.getenv('USERNAME') print(f'Your username is {username}')
Here’s another example—a similar program in another popular programming language, Go, which also has a package called “os” that is used to access environment variables and other operating system functionality:
package main import ( "fmt" "os" ) func printUsername() { username := os.Getenv("USERNAME") fmt.Printf("Your username is %s", username) }
Security Considerations
An important reason for using environment variables is to keep sensitive data like passwords away from source code and its repositories. However, it is easy for environment variables to become a security weakness themselves, because they are global in nature. They should thus be used carefully.
However, simple precautions can help mitigate the risks: The number of users who have access to the values should be limited to a bare minimum; files with variables should be kept away from common repositories. While these and similar measures seem trivial, they can make a real difference to security.
Using Environment Variables with Gcore Functions
Gcore’s Function as a service (FaaS) also supports environment variables. To use the feature, you create a namespace; while doing this you can define environment variables that will be available to all functions which belong to this namespace:
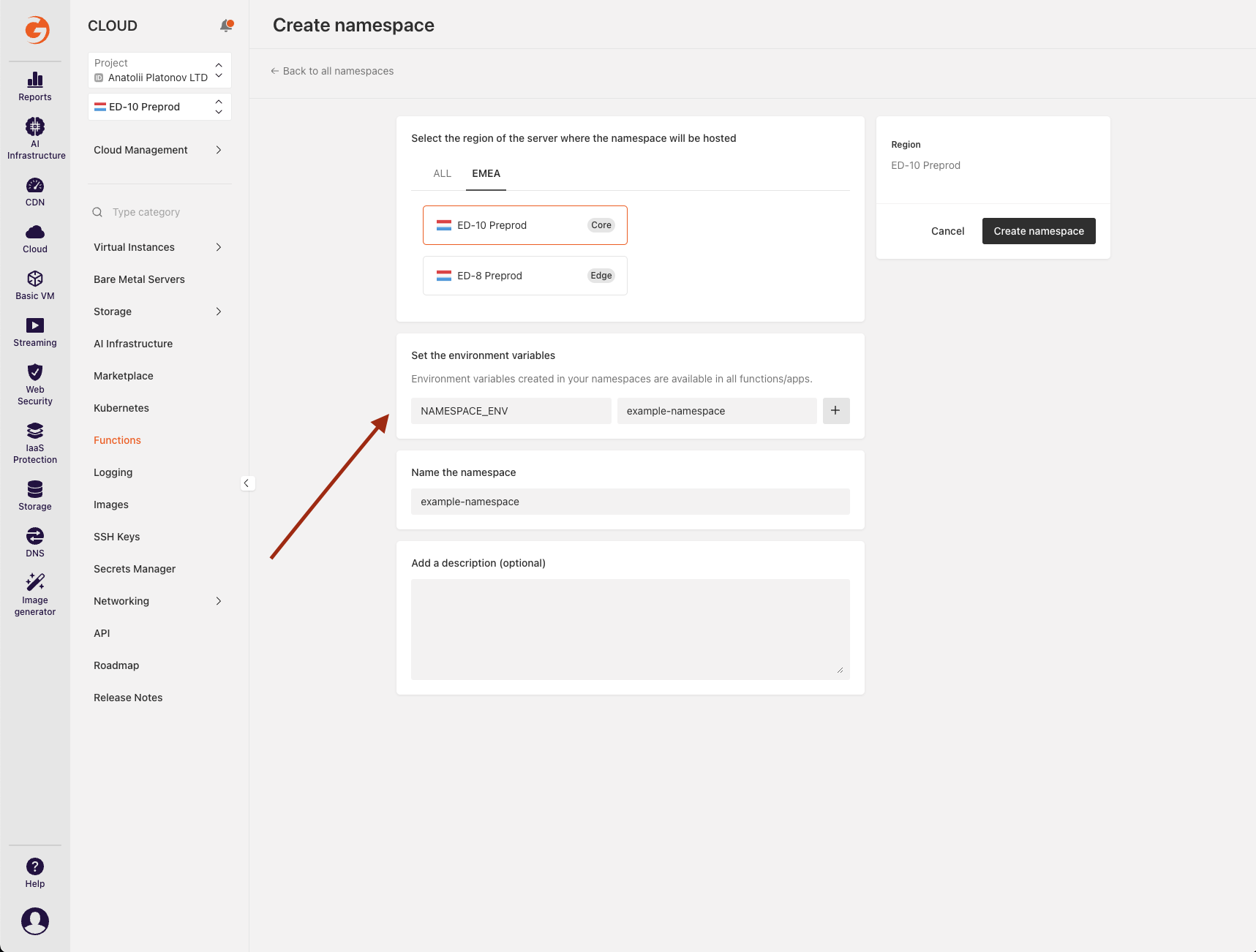
It’s also possible to declare more restricted environment variables which are only available to a single function. This may come in handy for specifying input parameters of the function, and thus decoupling configuration from code. This can be done while creating the function:
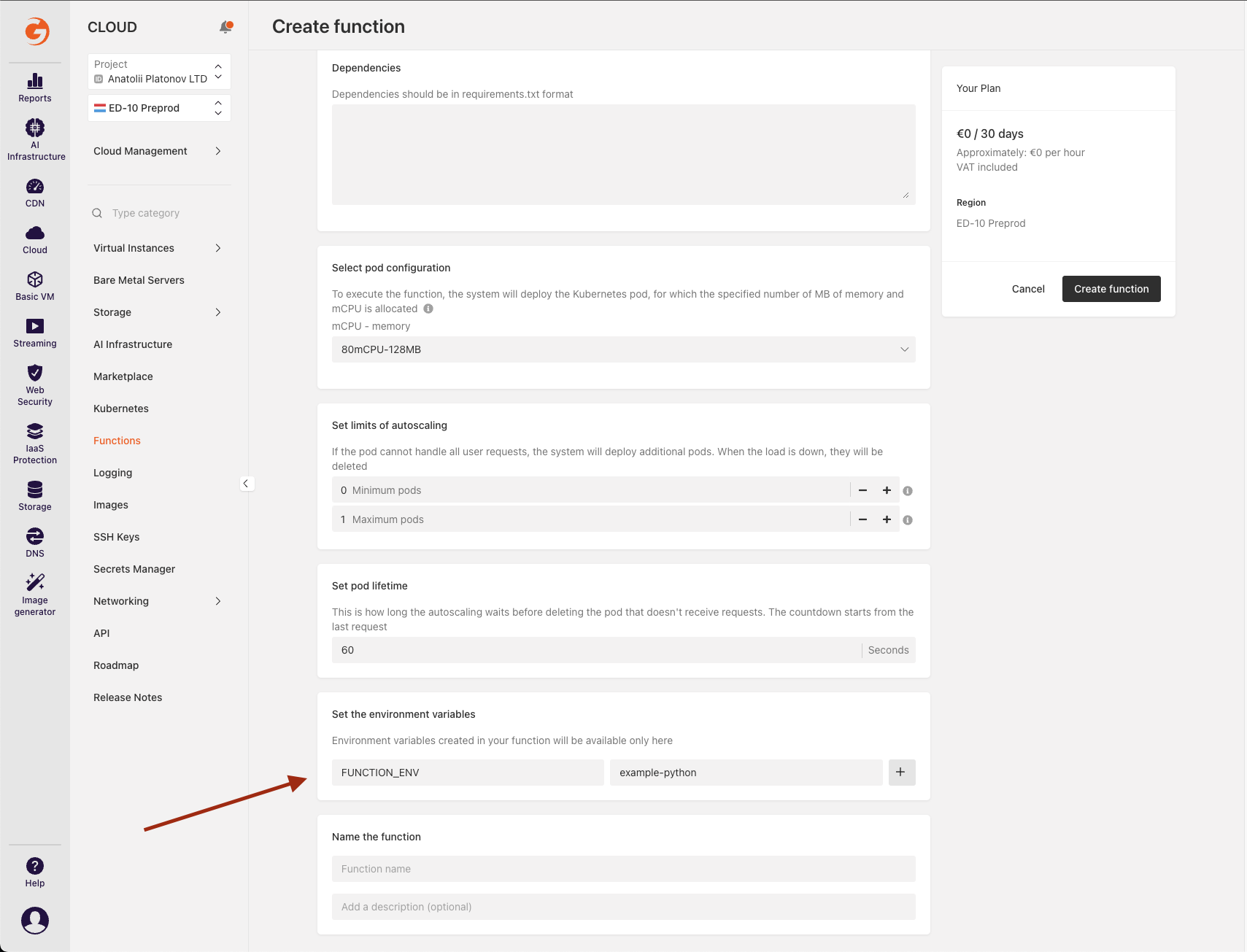
Conclusion
Environment variables are set by the user or provided by the operating system. They store values available to all scripts, programs, and functions within the scope of the variable. They are useful when input data is shared between multiple applications, or when it is preferable to extract data from the app’s source code and store it separately.
We also saw that environment variables can be used in platforms that provide FaaS. For example, in Gcore’s functions you can specify variables that are either available to all functions in a namespace, or as input parameters to a single function. If you need more information about the service, you can find it on the website or by reaching out to our support team.