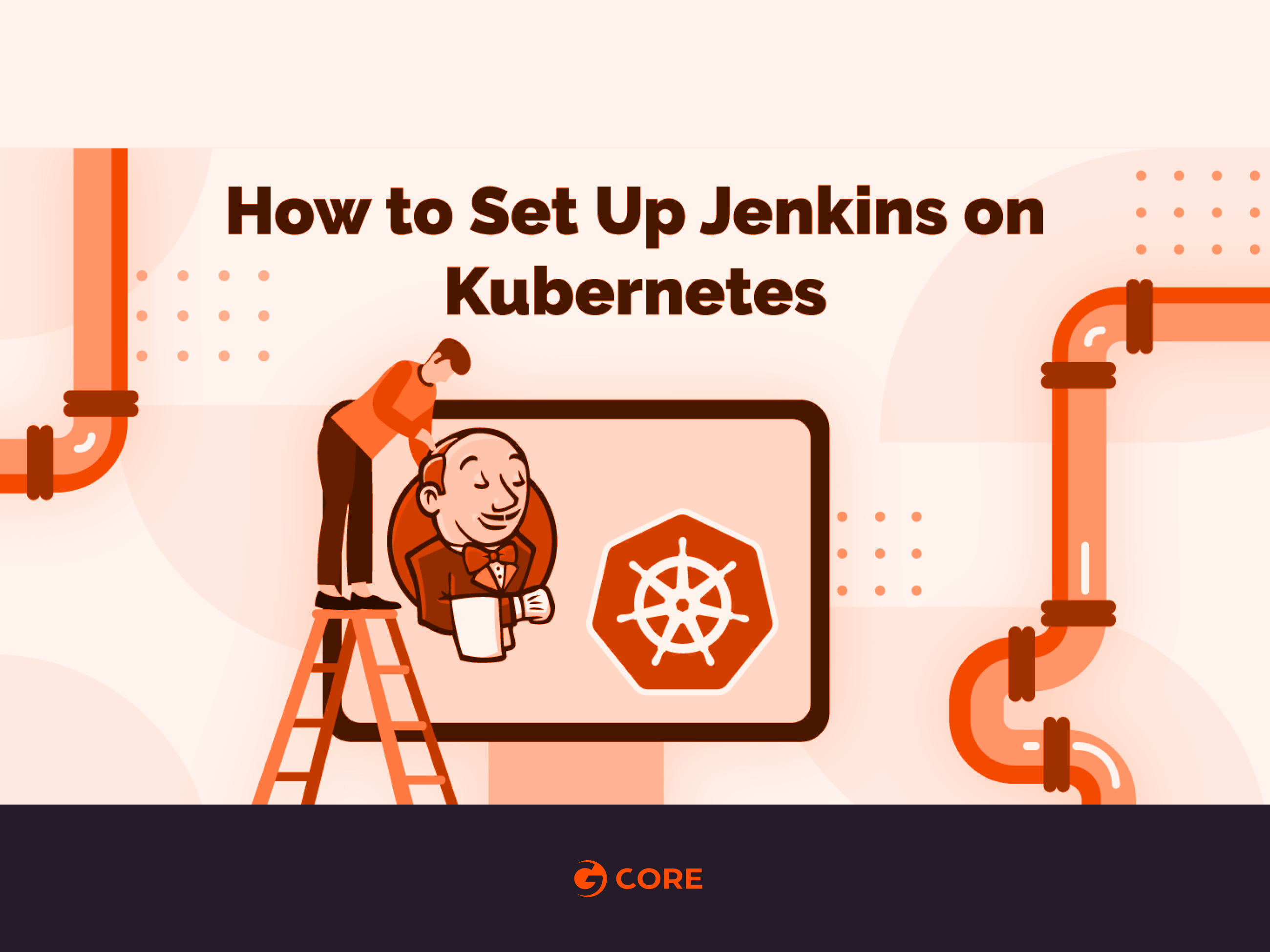
This guide will walk you through the process of setting up Jenkins on Kubernetes. Jenkins is a widely-used open source CI server that provides hundreds of plugins to support building, deploying and automating your projects.
In the next sections, you will:
- Create a Kubernetes cluster with minikube (This is an optional step. If you already have a running Kubernetes cluster, then you can skip this step)
- Create a namespace and a PersistentVolume for Jenkins
- Install Jenkins using Helm
- Set up and run a pipeline that tests a simple web application
Prerequisites
- Docker. For details about installing Docker, refer to the Install Docker page.
- A Kubernetes cluster. If you donât have a running Kubernetes cluster, see the âCreate a Kubernetes Cluster with minikubeâ section.
- Helm CLI. To install Helm CLI, follow the instructions from the Installing Helm page.
Create a Kubernetes Cluster with minikube (Optional)
Minikube is a tool that creates a single-node Kubernetes cluster on your computer. Follow these steps if you don’t have a running Kubernetes cluster:
- Install
minikube
by following the steps from the Install minikube page - Install
kubectl
. See the instructions from the Install and Set Up kubectl page. - You can now create a minikube cluster by entering the following command:
minikube start
đ minikube v1.5.2 on Darwin 10.15.2 âš Automatically selected the 'hyperkit' driver (alternates: [virtualbox]) đ„ Creating hyperkit VM (CPUs=2, Memory=2000MB, Disk=20000MB) ... đł Preparing Kubernetes v1.16.2 on Docker '18.09.9' ... đ Pulling images ... đ Launching Kubernetes ... â Waiting for: apiserver đ Done! kubectl is now configured to use "minikube"
- Once the cluster has been created, you can verify its status by entering:
minikube status
If all is going well, you should see something similar to this:
host: Running kubelet: Running apiserver: Running kubeconfig: Configured
Note that you can also get an overview of your cluster by using the built-in Kubernetes user interface. You can access it by running the following command:
minikube dashboard
Verifying dashboard health ... đ Launching proxy ... đ€ Verifying proxy health ... đ Opening http://127.0.0.1:56993/api/v1/namespaces/kubernetes-dashboard/services/proxy/ in your default browser...
This opens a web page providing information on the state of your Kubernetes cluster:
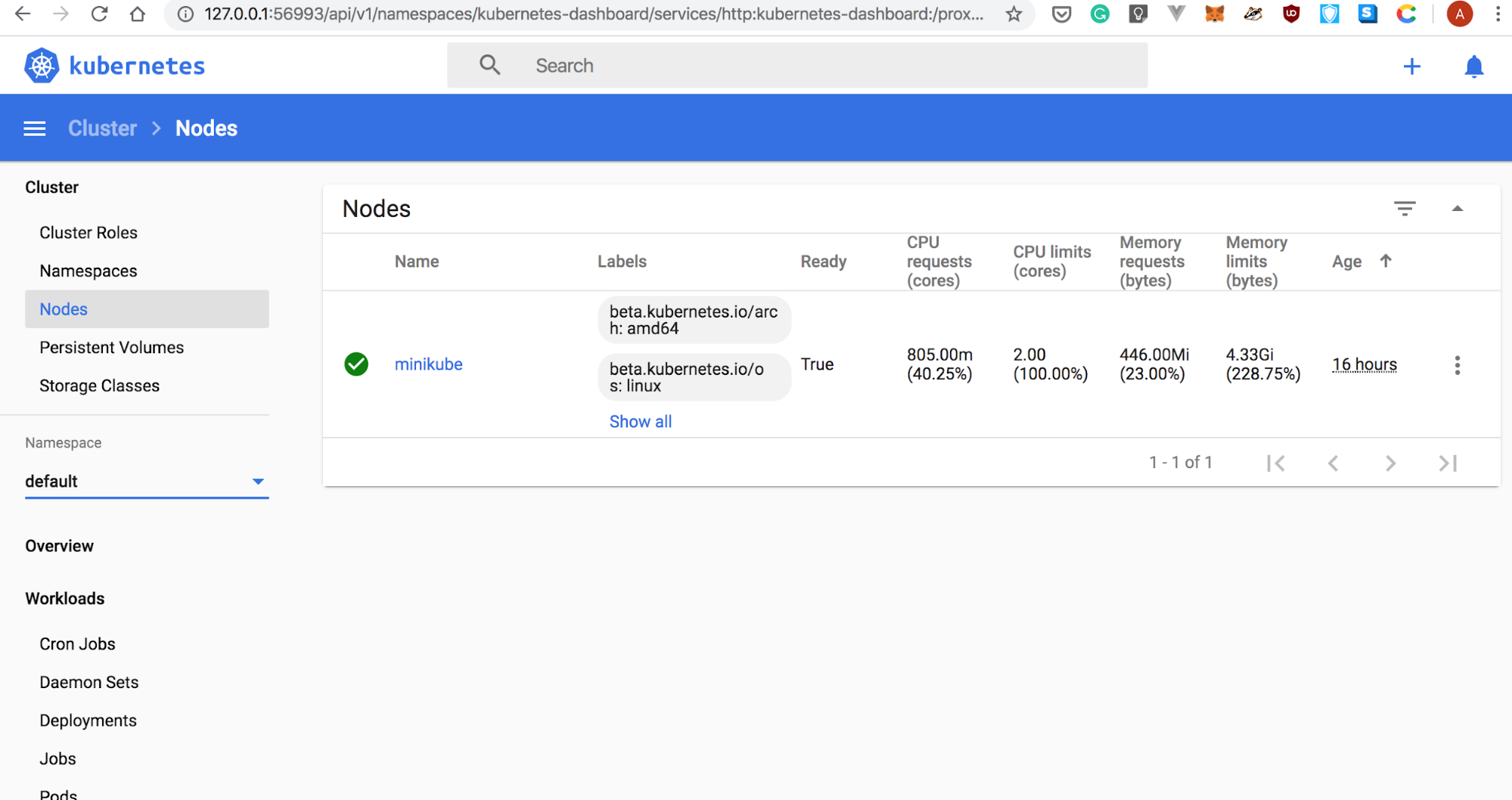
You can also use the dashboard to interact with your Kubernetes cluster. However, this is beyond the scope of this tutorial. If youâre inclined to learn more about creating or modifying Kubernetes resources using the Kubernetes dashboard, please refer to the Web UI (Dashboard) page.
Getting Set Up for Jenkins
To set up Jenkins you will:
- Create a namespace that allows you to segregate Jenkins objects within the Kubernetes cluster.
- Create a PersistentVolume to store your Jenkins data. This will preserve data across restarts.
Hereâs how you can get your environment set up for Jenkins:
- Create a file called
jenkins-namespace.yaml
with the following content:
apiVersion: v1 kind: Namespace metadata: name: jenkins
- Apply the spec by entering:
kubectl apply -f jenkins-namespace.yaml
namespace/jenkins created
- Paste the following snippet into a file called
jenkins-volume.yaml
:
apiVersion: v1 kind: PersistentVolume metadata: name: jenkins-pv namespace: jenkins spec: storageClassName: jenkins-pv accessModes: - ReadWriteOnce capacity: storage: 10Gi persistentVolumeReclaimPolicy: Retain hostPath: path: /data/jenkins-volume/
- Run the following command to apply the spec:
kubectl apply -f jenkins.volume.yaml
Itâs worth noting that, in the above spec, hostPath uses the
/data/jenkins-volume/
of your node to emulate network-attached storage. This approach is only suited for development and testing purposes. For production, you should provide a network resource like a Google Compute Engine persistent disk, or an Amazon Elastic Block Store volume.
Install Jenkins
A typical Jenkins deployment is comprised of a master node and, optionally, one or more agents. Jenkins is a complex application that relies on several components and, to simplify the deployment, youâll use Helm to deploy Jenkins. Helm is a package manager for Kubernetes and its package format is called a chart. Many community-developed charts are available on GitHub.
- To enable persistence, you will create an override file and pass it as an argument to the Helm CLI. Paste the content from https://raw.githubusercontent.com/kubernetes/charts/master/stable/jenkins/values.yaml into a file called
values.yaml
. Then, open thevalues.yaml
file in your favourite text editor and modify the following line:
storageClass:
to:
storageClass: jenkins-pv
- Now you can install Jenkins by running the
helm install
command and passing it the following arguments:
- The name of the release (
jenkins
) - The
-f
flag with the name of the YAML file with overrides (values.yaml
) - The name of the chart (
stable-jenkins
) - The
--namespace
flag with the name of your namespace (jenkins
)
helm install jenkins -f values.yaml stable/jenkins --namespace jenkins
This outputs something similar to the following:
NAME: jenkins LAST DEPLOYED: Mon Dec 30 17:26:08 2019 NAMESPACE: jenkins STATUS: deployed REVISION: 1 NOTES: 1. Get your 'admin' user password by running: printf $(kubectl get secret --namespace jenkins jenkins -o jsonpath="{.data.jenkins-admin-password}" | base64 --decode);echo 2. Get the Jenkins URL to visit by running these commands in the same shell: export POD_NAME=$(kubectl get pods --namespace jenkins -l "app.kubernetes.io/component=jenkins-master" -l "app.kubernetes.io/instance=jenkins" -o jsonpath="{.items[0].metadata.name}") echo http://127.0.0.1:8080 kubectl --namespace jenkins port-forward $POD_NAME 8080:8080 3. Login with the password from step 1 and the username: admin
- Depending on your environment, it can take a bit of time for Jenkins to start up. Enter the following command to inspect the status of your Pod:
kubectl get pods --namespace=jenkins
Once Jenkins is installed, the status should be set to Running
as in the following output:
⯠kubectl get pods --namespace=jenkins NAME READY STATUS RESTARTS AGE jenkins-645fbf58d6-6xfvj 1/1 Running 0 2m
- To access your Jenkins server, you must retrieve the password by entering the following command:
printf $(kubectl get secret --namespace jenkins jenkins -o jsonpath="{.data.jenkins-admin-password}" | base64 --decode);echo
Um1kJLOWQY
đđ» Note that your password will be different.
- Get the name of the Pod running that is running Jenkins using the following command :
export POD_NAME=$(kubectl get pods --namespace jenkins -l "app.kubernetes.io/component=jenkins-master" -l "app.kubernetes.io/instance=jenkins" -o jsonpath="{.items[0].metadata.name}")
This will create an environment variable called POD_NAME
and set its value to the name of the Pod that is running Jenkins.
- Use the
kubectl
command to set up port forwarding:
kubectl --namespace jenkins port-forward $POD_NAME 8080:8080
Forwarding from 127.0.0.1:8080 -> 8080 Forwarding from [::1]:8080 -> 8080
Add an Executor
The Jenkins documentation defines an executor as âA slot for execution of work defined by a Pipeline or Project on a Node. A Node may have zero or more Executors configured which corresponds to how many concurrent Projects or Pipelines are able to execute on that Node.â In this section, you will add an executor to your Jenkins node.
- Point your browser to
http://localhost:8080
and log in using theadmin
username and the password you retrieved earlier - In the left-hand column, go to Jenkins -> Manage Jenkins -> Manage Node
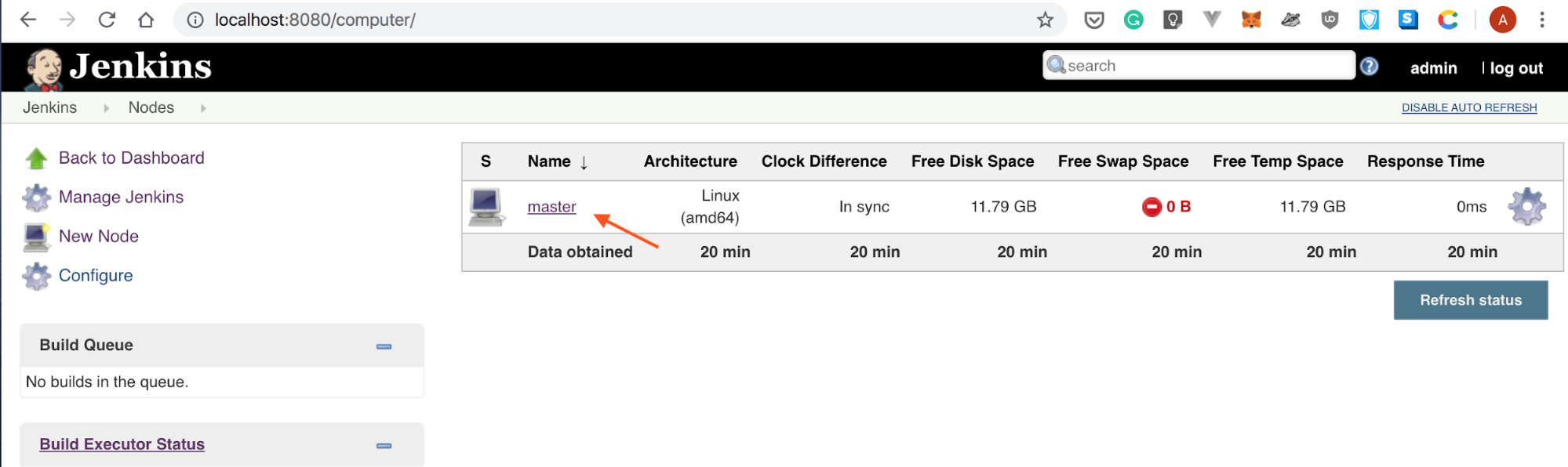
- Select Master and then click Configure
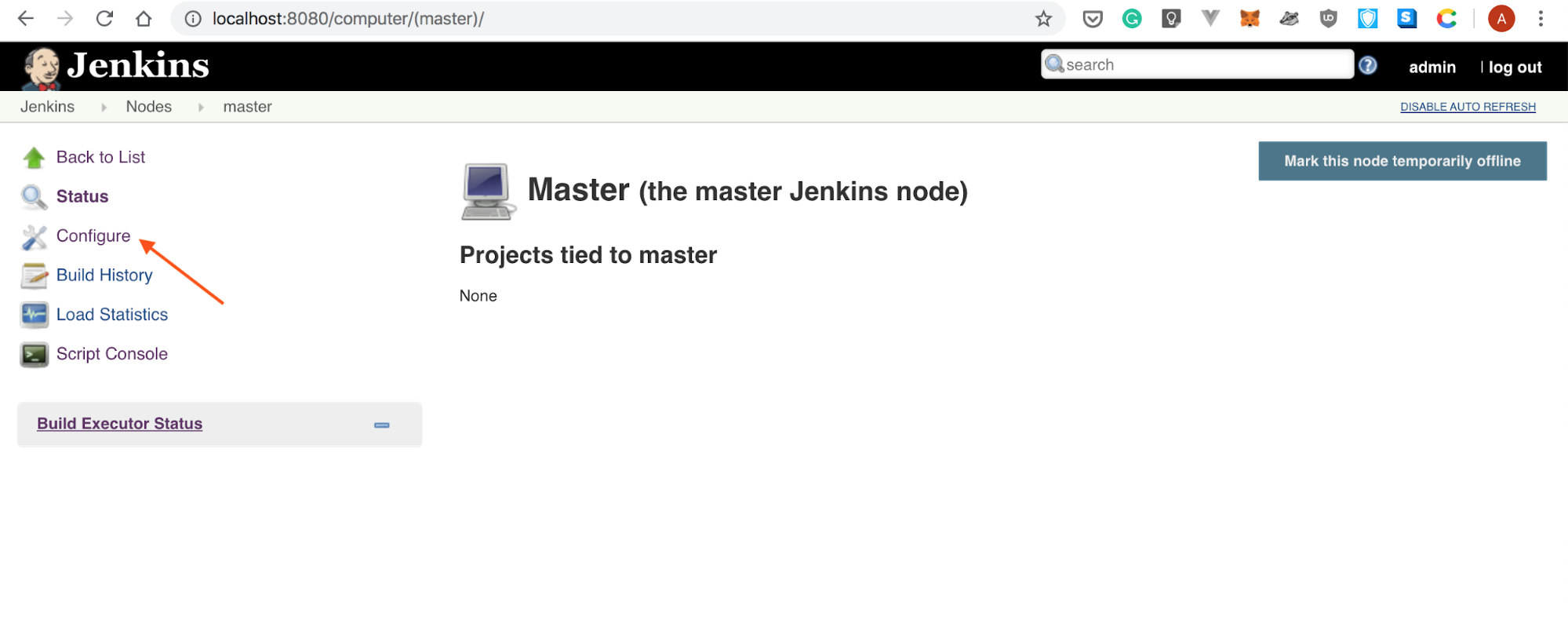
- Type â1â in the # of executors input box and then click the Save button
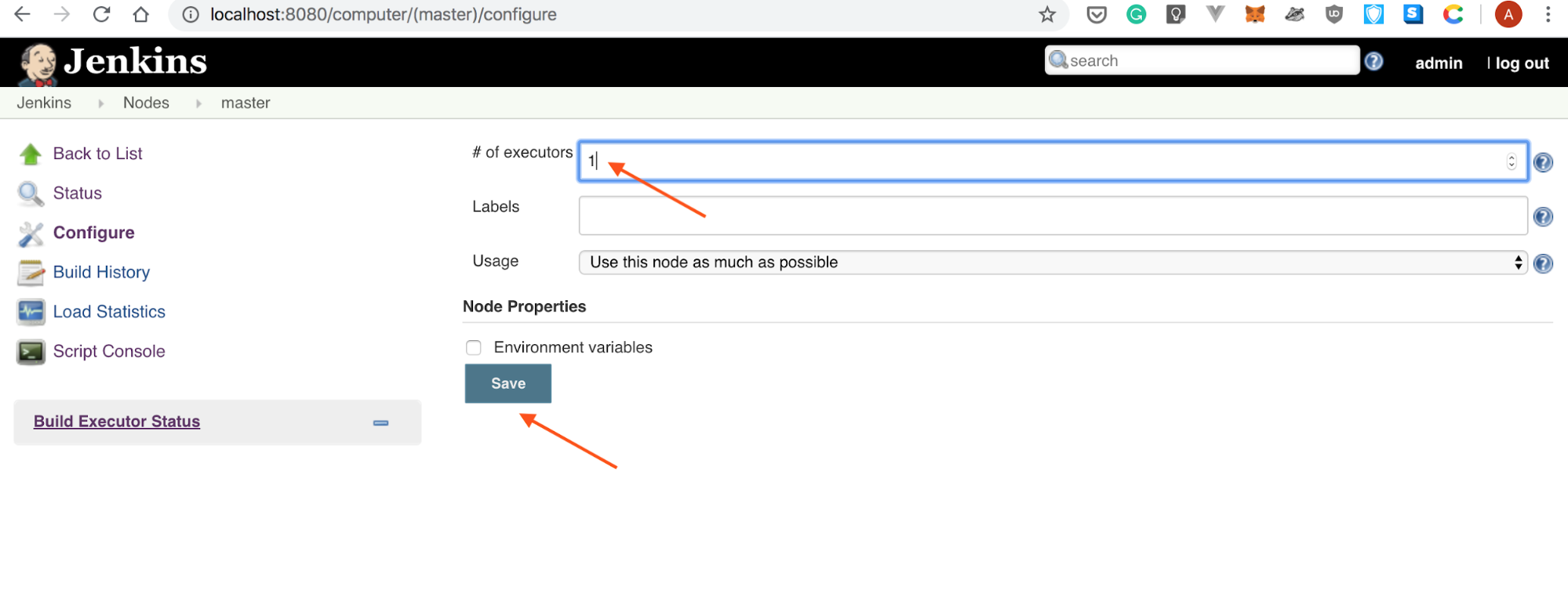
Install and Configure the Node.js Plugin
The Node.js plugin integrates Jenkins with Node.js and, for the scope of this tutorial, youâll use this plugin to deploy and test a simple web application written in Express.js.
Follow these steps to install the Node.js plugin:
- In the left-hand column, go to Jenkins -> Manage Manage Jenkins -> Manage Plugins -> Available Plugins and select the NodeJS plugin. Then, click the Install without restart button.
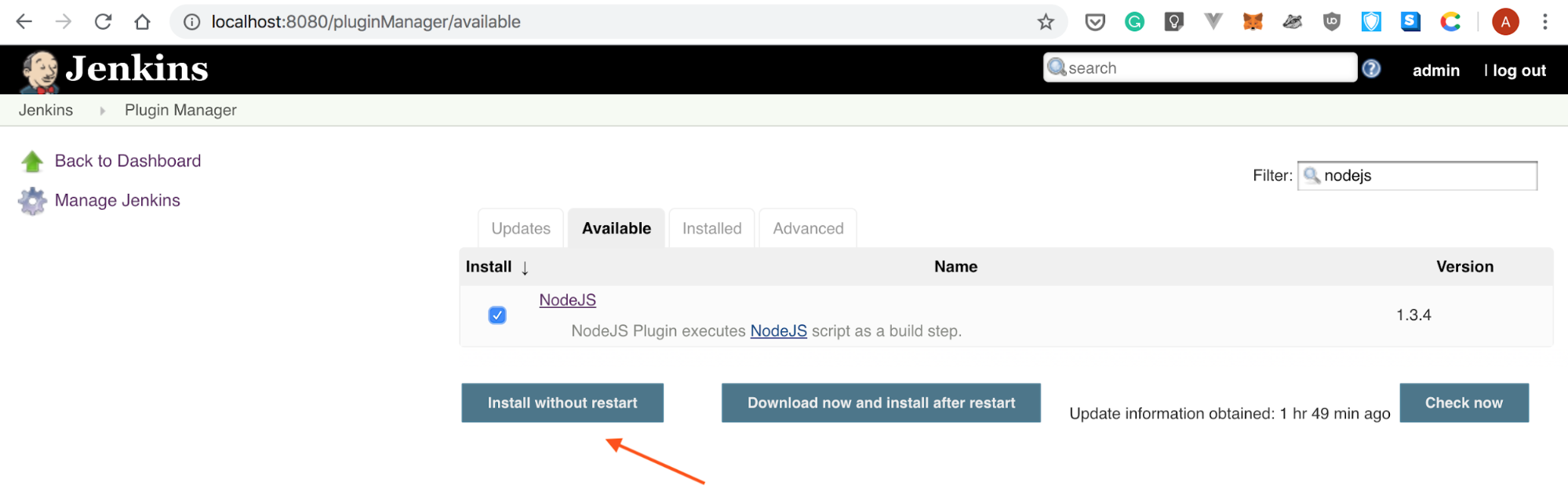
- Once the installation is finished, youâll see something like the following:
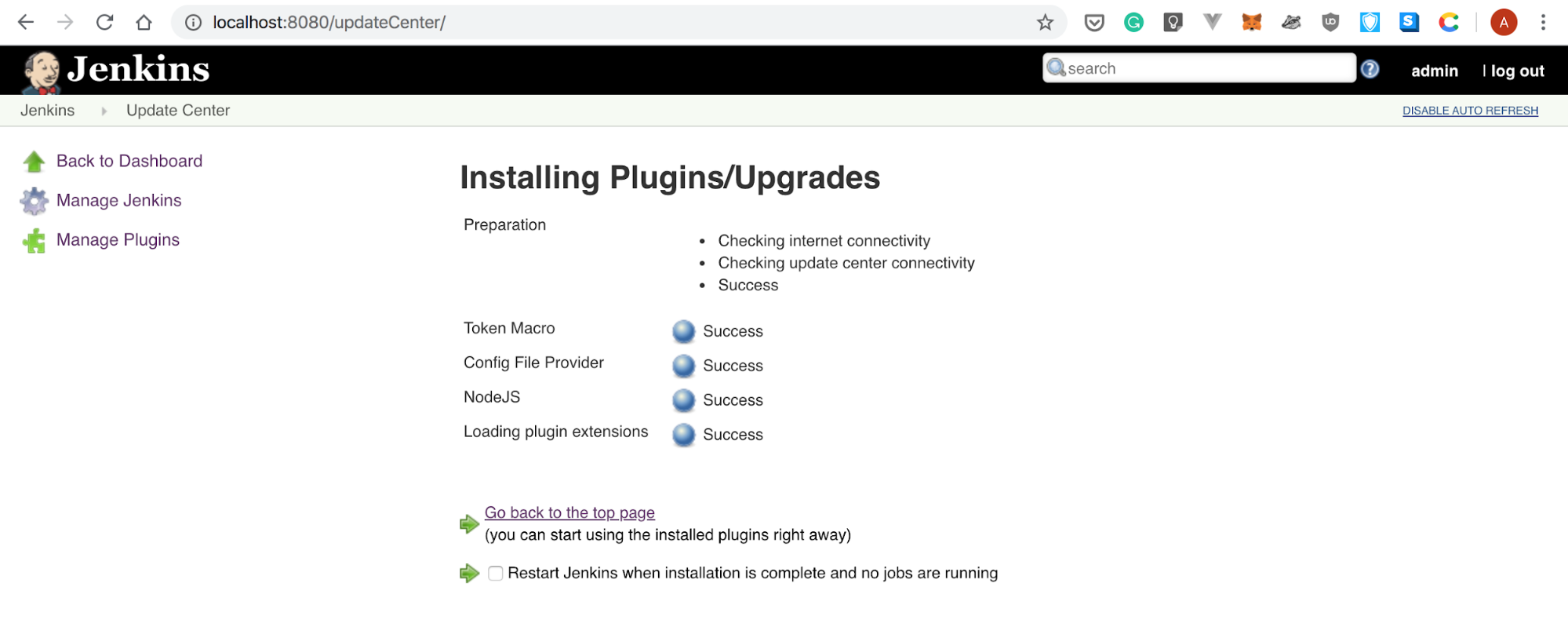
- In the left-hand column, go to Jenkins -> Manage Jenkins -> Global Tool Configuration and select Add NodeJS. Type the name of your NodeJS installation, and then choose a NodeJS. version. Once youâre done, click the Save button
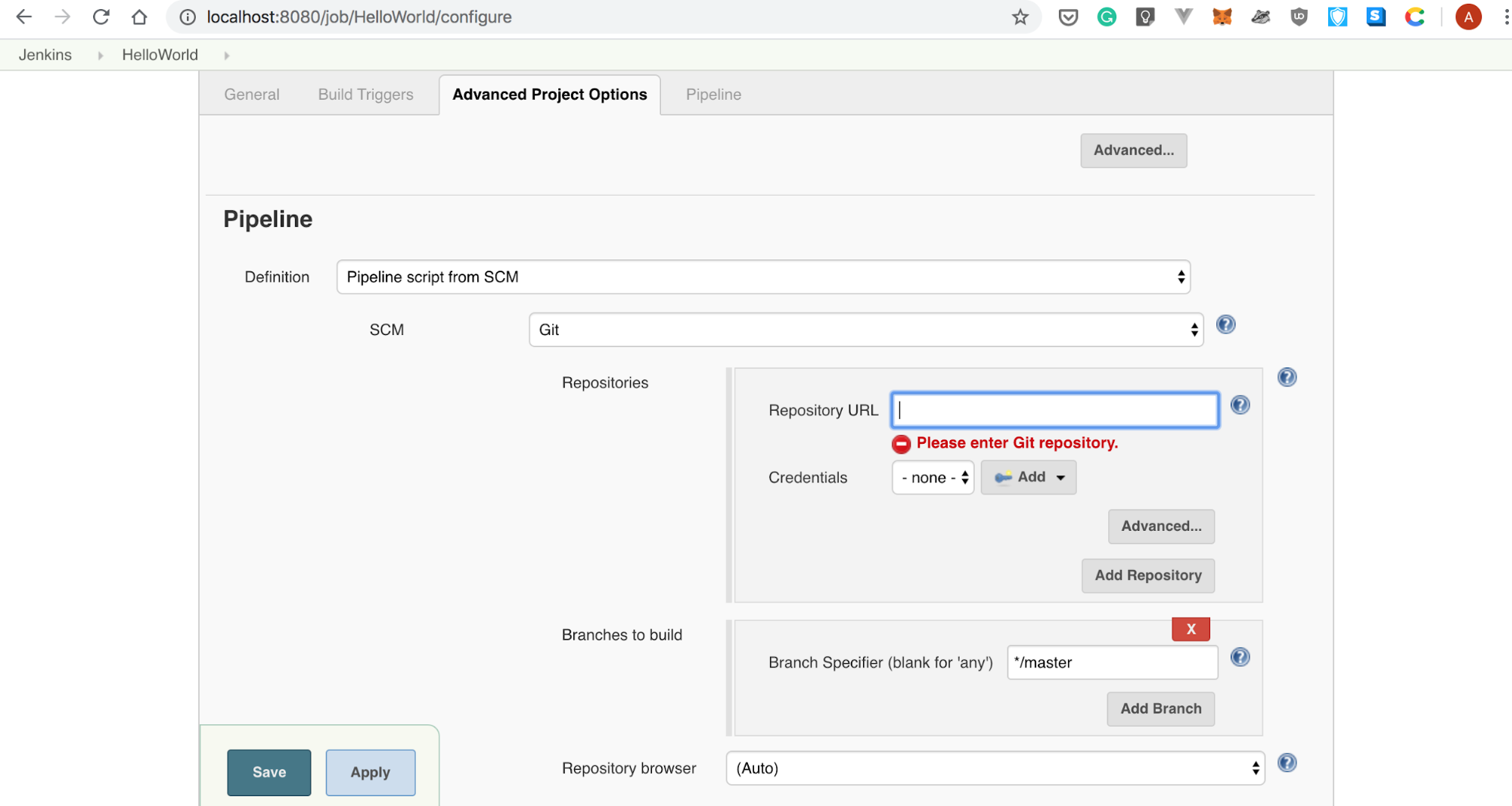
The HelloWorld Repository
To showcase how Jenkins works, we created a simple Express.js server that listens on port 3000 and prints âHello World!â. Then, we wrote a unit test that checks if the web application works as expected. We also updated the package.json file so that Jenkins can execute the unit test with the npm test
command. Lastly, we created a Jenkinsfile
with the following content:
pipeline { agent any tools {nodejs "node"} stages { stage('Cloning Git') { steps { git 'https://github.com/andreipope/HelloWorld' } } stage('Install dependencies') { steps { sh 'npm install' } } stage('Test') { steps { sh 'npm test' } } } }
The above listing defines a three-stage CD pipeline:
- First, it clones the HelloWorld Git repository
- Second, it installs the dependencies by running the
npm install
- Lastly, it executes the unit test.
In the next section, youâll configure Jenkins to run this script.
Create and Run a Pipeline
A pipeline provides a repeatable and consistent process for delivering software. With Jenkins, you can write your pipeline as a DSL script based on the Groovy programming language.
Follow these steps to create and run the pipeline:
- Go to Jenkins -> New Item and enter the name of the pipeline (
HelloWorld
). Once youâre done, click the OK button.
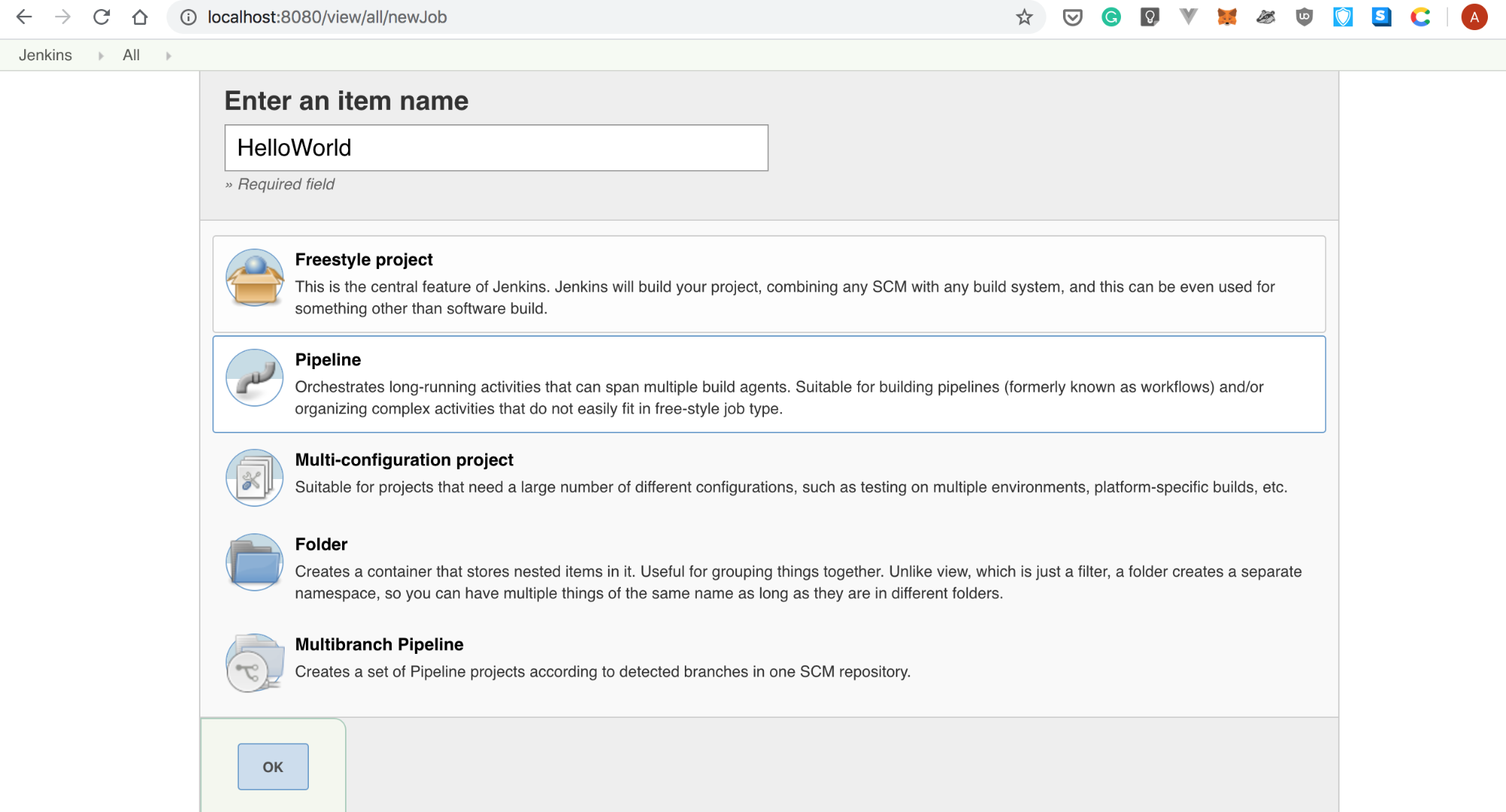
- On the next page, select Pipeline script from SCM and set the Repository URL to “https://github.com/andreipope/HelloWorld”. This is a public repository, meaning that you donât need to configure any credentials.
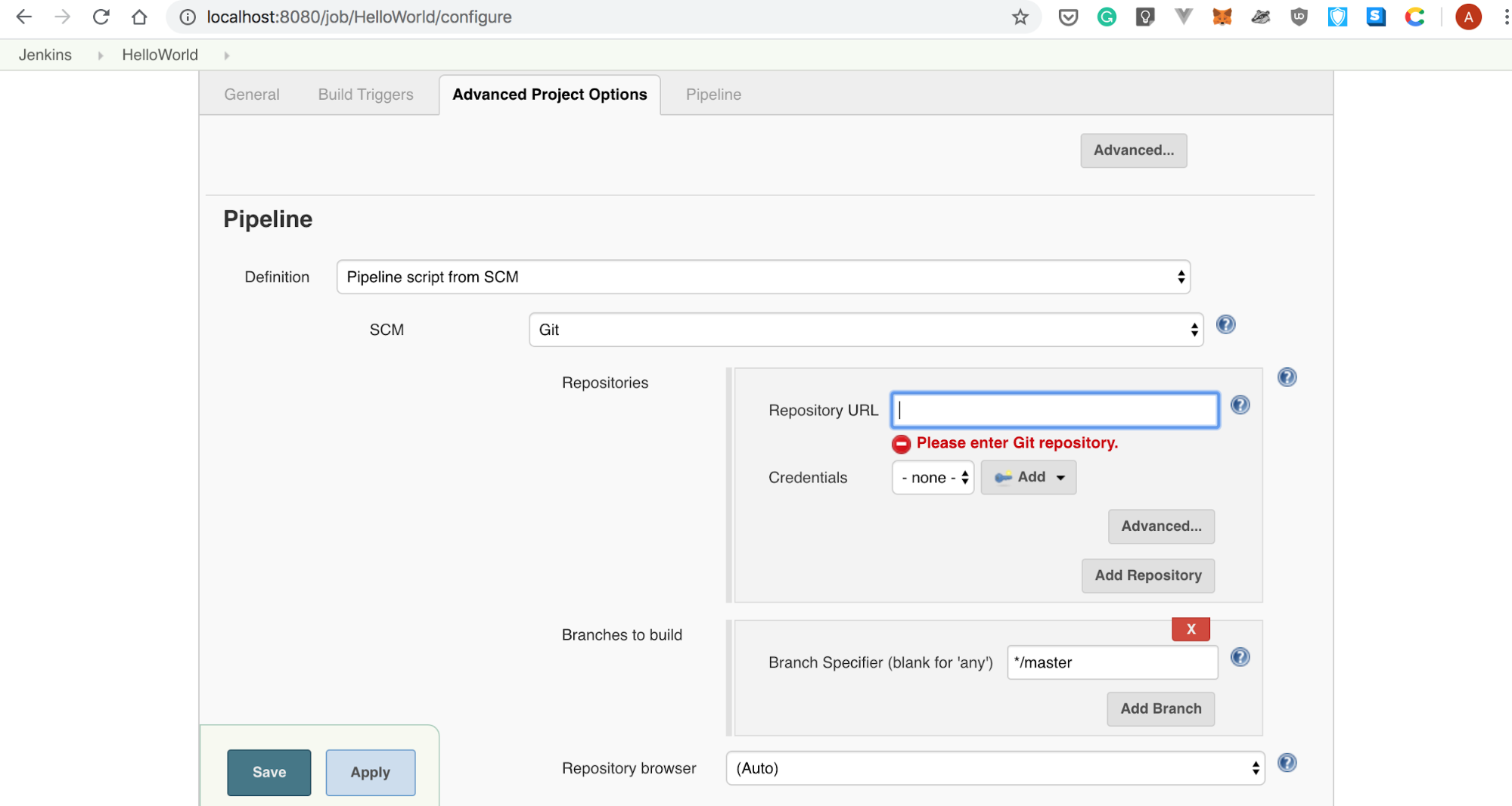
Jenkins will automatically retrieve the
Jenkinsfile
located in the root folder of the repository.
- Now you can manually run the pipeline:
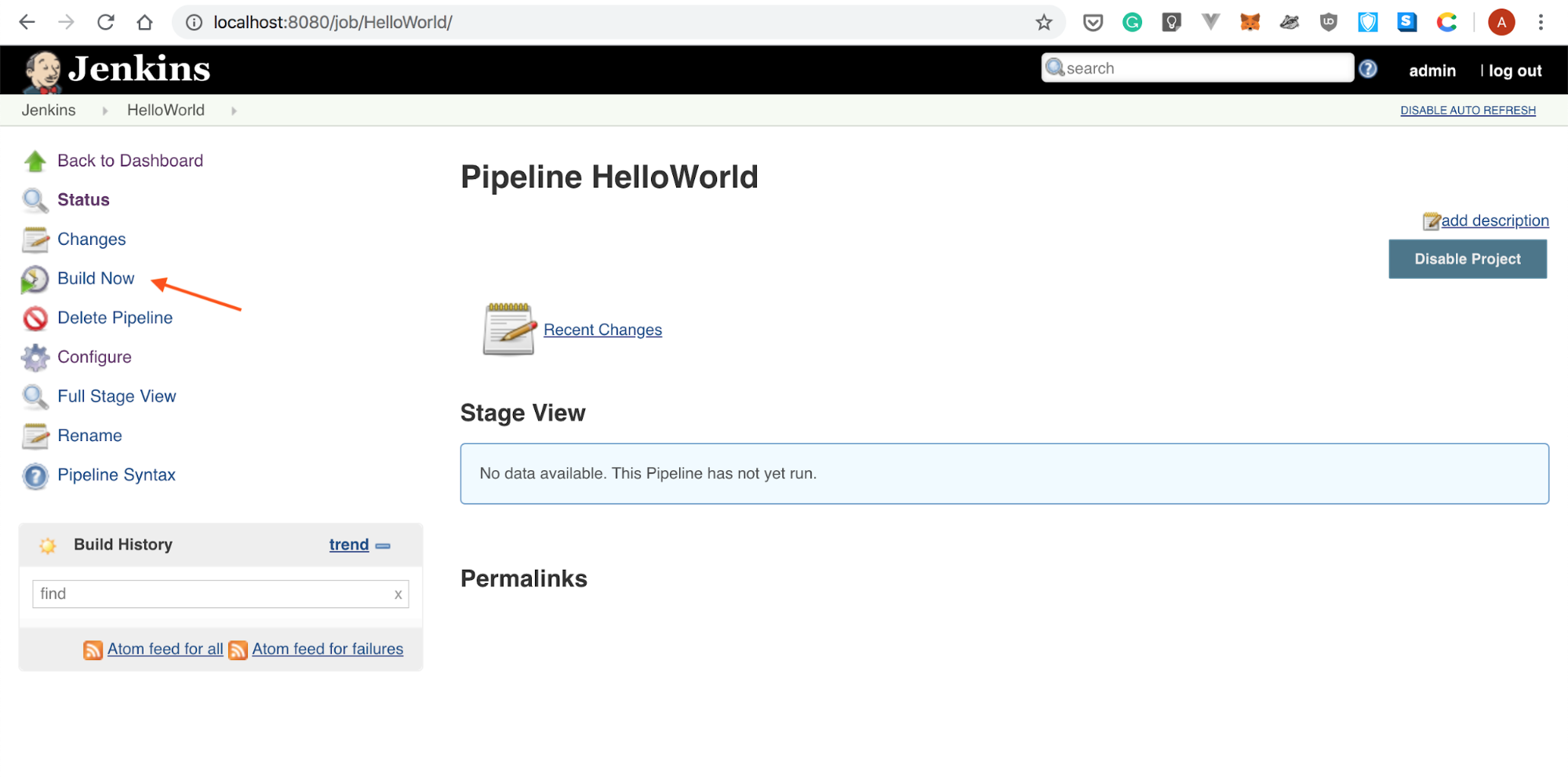
- Give it some time to run. Once the process is finished, you should see something like the following printed out to the console:
+ npm test > HelloWorld@1.0.0 test /var/jenkins_home/workspace/HelloWorld > mocha ExpressJS server â Prints out Hello World (291ms) 1 passing (373ms) [Pipeline] } [Pipeline] // withEnv [Pipeline] } [Pipeline] // stage [Pipeline] } [Pipeline] // withEnv [Pipeline] } [Pipeline] // withEnv [Pipeline] } [Pipeline] // node [Pipeline] End of Pipeline Finished: SUCCESS
Congratulations! Youâve set up Jenkins with Kubernetes and then used it to test a simple web application. Even if you might feel like youâve covered a lot of ground, the truth is youâve only got your feet wet with what Jenkins and Kubernetes can do! In future tutorials, we’ll walk through examples of more advanced use cases.